How to create Laravel localization
Today I will give you an example of how to create Laravel localization. In this example, you can understand the concept of Laravel multilingual websites and also gain knowledge about how to add multiple languages in Laravel. a simple example of Laravel multi-language with a different list of language dropdowns. we will use Laravel default function trans() to create a Multilingual language website.
Laravel localization features provide a convenient way to retrieve text in different languages, Like China, India, etc. So I will show you how to create localization or Laravel dynamic language.
Install Laravel
We are a scratch new project setup for this example, So create a new project using the below command laravel/laravel
.
composer create-project --prefer-dist laravel/laravel blog
Create Localization File
We will Create a localization file language-wise. you copy and paste the below code custom message or value.
# resources/lang/en/messages.php <?php return [ 'title' => 'How to create a localization!', ];
# resources/lang/zhh/messages.php <?php return [ 'title' => '如何创建本地化!', ];
Add Route
Now, we will create two routes first is a view page with a language dropdown and message and the second is changing languages using jQuery.
# routes/web.php Route::get('index', 'LaravelLocalizationController@index'); Route::get('change_lang', 'LaravelLocalizationController@change_lang')->name('ChangeLang');
Create Controller
We create a new controller as a LaravelLocalizationController. This controller helps to manage layouts and change language dynamically using the dropdown.
php artisan make:controller LaravelLocalizationController
Now, add below code in your LaravelLocalizationController
.
# app/Http/Controllers/LaravelLocalizationController.php <?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App; class LaravelLocalizationController extends Controller { public function index() { return view('language'); } public function change_lang(Request $request) { App::setLocale($request->lang); session()->put('lang', $request->lang); return view('language'); } }
Create Blade File
We will create a blade file to view our output. and copy the below code and paste in the blade file.
# resources/views/language.blade.php <!DOCTYPE html> <html> <head> <title>How to create laravel localization - devnote.in</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initialscale=1"> <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css" rel="stylesheet"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> </head> <body> <div class="container"> <div class="row" style="text-align: center;margin-top: 20px;"> <h2>How to create laravel localization - devnote.in</h2><br> <div class="col-md-4 col-md-offset-3 text-right"> <strong>Select Language: </strong> </div> <div class="col-md-4"> <select class="form-control change_lang"> <option value="en" {{ session()->get('lang')== 'en' ? 'selected' : '' }}>English</option> <option value="zhh" {{ session()->get('lang') == 'zhh' ? 'selected' : '' }}>Chinese</option> </select> </div> <br><br> <h1>{{ __('messages.title')}}</h1> </div> </div> </body> <script type="text/javascript"> var url = "{{ route('ChangeLang') }}"; $(".change_lang").change(function(){ window.location.href = url + "?lang="+ $(this).val(); }); </script> </html>
Laravel localization provides a display string or message using different ways Like.
trans()
@lang()
__()
@json(__())
Run this example in your browser.
http://localhost:8000/index
English Language
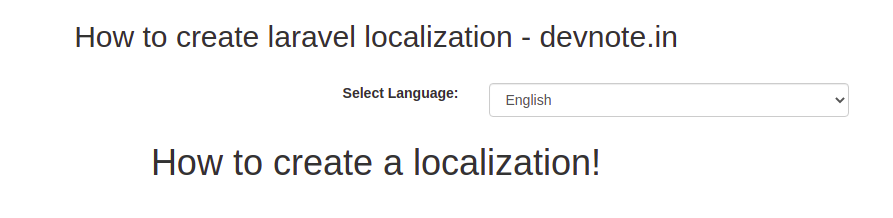
Chinese Language
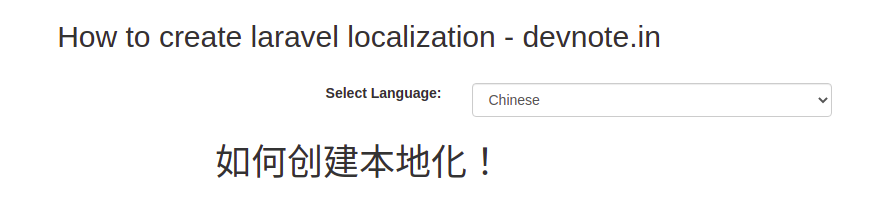