How to integrate Google reCAPTCHA in Laravel
This tutorial is on How to integrate Google reCAPTCHA in Laravel. Google Recaptcha is used to advance risk analysis techniques to show humans and bots apart. Google reCAPTCHA works on the basis of spam score and the reCAPTCHA API returns the spam score of each request given by the user activity. this tutorial is on how to add Google reCAPTCHA to a form on a Laravel website. we are using anhskohbo/no-captcha Laravel package. And most important we need a secret key and site key from Google API.
Install Laravel
First, install fresh Laravel Type the following command in the terminal.
composer create-project --prefer-dist laravel/laravel google_recaptcha
Install anhskohbo/no-captcha Package
After the successful installation of the project, we need to install anhskohbo/no-captcha Package.
composer require anhskohbo/no-captcha
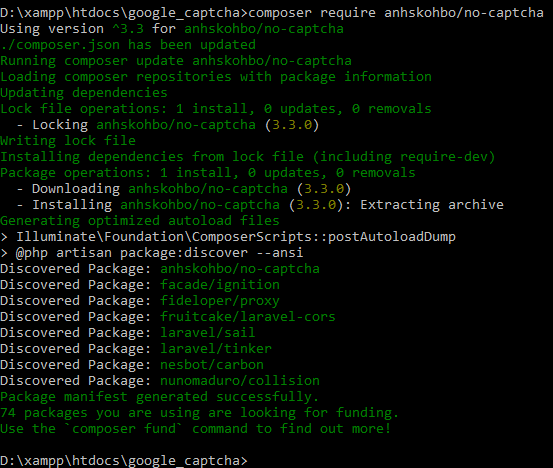
Publish Config File
Successfully install anhskohbo/no-captcha
package then publish config file.
php artisan vendor:publish --provider="Anhskohbo\NoCaptcha\NoCaptchaServiceProvider"
Output: Copied File [\vendor\anhskohbo\no-captcha\src\config\captcha.php] To [\config\captcha.php] Publishing complete.
Add Google Site Key and Secret Key
We need to add Google Site Key and Secret Key in the .env file, and if you
don’t have these keys then you need to create them from google’s website.
Also read: How to integrate Google reCAPTCHA v3 in PHP
Now open the .env file and add these keys.
# .env
NOCAPTCHA_SECRET=put_your_secret_key
NOCAPTCHA_SITEKEY=put_your_site_key
Create Controller
Now create a controller on this path app\Http\Controllers\UserController.php and add the below command.
php artisan make:controller UserController -r
<?php # app\Http\Controllers\UserController.php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\User; class UserController extends Controller { public function create() { return view('create_user'); } public function store(Request $request) { $request->validate([ 'name' => 'required', 'email' => 'required|email', 'g-recaptcha-response' => 'required|captcha', ]); User::create($request->all()); return "Successfully created!"; } }
Add Route
# routes/web.php Route::resource('users',UserController::class);
Create Blade File
Now create a create_user.blade.php file in this path resources\views\create_user.blade.php and put the below HTML code.
resources\views\create_user.blade.php
<html>
<head>
<title>How to integrate Google reCAPTCHA in Laravel - Devnote.in</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<style>
.form-group div div {
width: auto !important;
}
</style>
</head>
<body class="text-center">
<div class="container">
<h1>How to integrate Google reCAPTCHA in Laravel</h1>
<div class="row" style="margin-top: 10px;">
<div class="col-lg-12 margin-tb">
<div class="text-center">
<h2>Create User</h2>
</div>
</div>
</div>
@if ($errors->any())
<div class="alert alert-danger">
<strong>Errors!</strong> <br>
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
<form action="{{ route('users.store') }}" method="POST">
@csrf
<div class="align-center">
<div class="row">
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>User name :</strong>
<input type="text" name="name" class="formcontrol" placeholder="User name">
</div>
</div>
</div>
<div class="row">
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong> User email:</strong>
<input type="email" name="email" class="formcontrol" placeholder="User email">
</div>
</div>
</div>
<div class="row">
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Google recaptcha :</strong>
{!! NoCaptcha::renderJs() !!}
{!! NoCaptcha::display() !!}
</div>
</div>
</div>
<div class="row">
<div class="col-xs-12 col-sm-12 col-md-12">
<button type="submit" class="btn btnprimary">Submit</button>
</div>
</div>
</div>
</form>
</div>
</body>
</html>