How to upload image in Laravel 8
In this tutorial, I show How to upload an image in Laravel 8 and add validation in the Laravel 8 project.
Once the files are selected, we’ll start uploading them and check validation. With end-to-end encryption and a secure file stored in a folder. PHP file upload features allow you to upload both binary and text files both.PHP allows you to upload single and multiple files through a few lines of code only.
Contents
- Create controller
- Route define
- Create view
- Output
- Conclusion
1. Create a controller
Create a HomeController
controller. run the below command:
php artisan make:controller HomeController
Now, Create a controller we will create two methods
index()
– index viewupload_file()
– This method is used to upload the file.
Validation
- Upload file validation. I set the max upload file size to 1 MB (1024 KB).
- If the successfully upload file then assign the file name to
$file_name
and upload file location files to the store$location
variable. $file->move($location,$file_name);
to store the file.- We use SESSION flash to store and upload class names and messages.
- Redirect to ‘/’.
Example
<?php #app\Http\Controllers\HomeController.php namespace App\Http\Controllers; use Illuminate\Http\Request; use Session; use Validator; class HomeController extends Controller { public function index() { return view('index'); } public function upload_file(Request $request) { // Laravel validation $validator = Validator::make($request->all(), [ 'profile' => 'required|mimes:png,jpg,jpeg|max:1024' ]); if($validator->fails()) { return Redirect::back()->withErrors($validator); } if($request->has('profile')) { $upload_file = $request->file('profile'); $file_name = time().'_'.$upload_file->getClientOriginalName(); // Upload file location $location = 'user_profile'; // Upload file in folder $upload_file->move($location,$file_name); Session::flash('message','User profile successfully uploaded!.'); Session::flash('alert-class', 'alert-success'); } else { Session::flash('message','User profile not uploaded, Please try again!'); Session::flash('alert-class', 'alert-danger'); } return redirect('/'); } }
2. Route define
<?php #routes/web.php use Illuminate\Support\Facades\Route; use App\Http\Controllers\HomeController; Route::get('/', [HomeController::class, 'index']); Route::post('/upload_file', [HomeController::class, 'upload_file'])->name('upload_file');
3. Create view
create index.blade.php file in resources/views/.
#resources/views/index.blade.php
<!DOCTYPE html>
<html>
<head>
<title>How to upload image in laravel 8 - devnote.in</title>
<!-- Meta data -->
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<meta charset="utf-8">
<link rel="stylesheet" type="text/css" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
</head>
<body>
<h1 class="text-center">How to upload image in laravel 8 - devnote.in</h1>
<div class="container">
<div class="row">
<div class="col-md-12 col-sm-12 col-xs-12">
@if($errors->has('profile'))
<div class="alert alert-danger">
{{ $errors->first('profile') }}
</div>
@endif
@if(Session::has('message'))
<div class="alert {{ Session::get('alert-class') }}">
{{ Session::get('message') }}
</div>
@endif
<form action="{{route('upload_file')}}" enctype="multipart/form-data" method="post">
{{csrf_field()}}
<div class="form-group">
<label class="col-md-3 col-sm-3 col-xs-12 control-label" for="profile">User profile</label>
<div class="col-md-6 col-sm-6 col-xs-12">
<input type='file' name='profile' id="profile" class="form-control">
@if ($errors->has('profile'))
<span class="errormsg text-danger">{{ $errors->first('profile') }} </span>
@endif
</div>
<div class="col-md-3">
<input type="submit" name="submit" value="Submit" class="btn btn-success">
</div>
</div>
</form>
</div>
</div>
</div>
</body>
</html>
4. Output
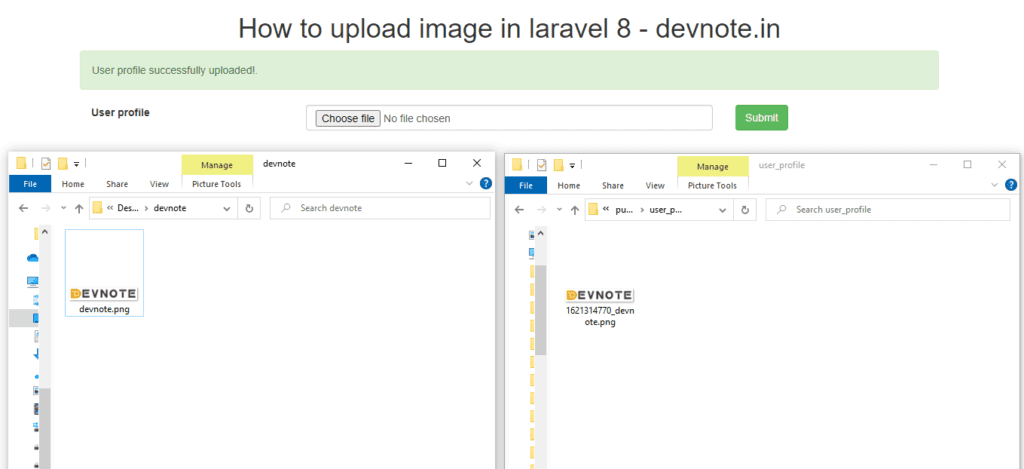
5. Conclusion
You can modify the validation according to your requirement in your controller. Folder created while uploading if not exists.
If you are allowed to upload large files then make sure to check
upload_max_filesize and post_max_size values in the php.ini(D:\xampp\php\php.ini) file and update.