How to create add-to-cart functionality in Laravel
In this tutorial, we will discuss How to create add-to-cart functionality in Laravel. Let’s discuss the Laravel add-to-cart session example. We will use Laravel eCommerce add to cart. So, follow a few steps to create an example of How to create add-to-cart functionality in Laravel. I will show you how to create add-to-cart functionality in Laravel step by step. Now we working with the eCommerce project then we must need to create add to cart function.
In this example, I will create a product table and display it in the list and you can add it to the cart that product. also, all framework cart pages where you can remove the product from there and change product quality.
- Install Laravel
- Create table
- Create Model
- Create Seeder
- Create Controller
- Define Route
- Create Blade Files
Install Laravel
First, we need to get Laravel 8 application using below command:
composer create-project --prefer-dist laravel/laravel blog
Create table
In this step, we need to create a products table using below command:
php artisan make:migration create_products_table
<?php #database\migrations\2021_06_27_053318_create_products_table.php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreateProductsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('products', function (Blueprint $table) { $table->id(); $table->string("name")->nullable(); //added $table->string("image")->nullable(); //added $table->decimal("price", 6, 2); //added $table->text("description")->nullable(); //added $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('products'); } }
Now run the bellow command to migration:
php artisan migrate
Create Model
In this step, we need to create a Product model using bellow command:
php artisan make:model Product
<?php #app\Models\Product.php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Product extends Model { use HasFactory; protected $fillable = ['name', 'image', 'price', 'description']; //added }
Create Seeder
Run the below command to create a Product seeder.
php artisan make:seed ProductSeeder
<?php #database\seeders\ProductSeeder.php namespace Database\Seeders; use Illuminate\Database\Seeder; use App\Models\Product; //added class ProductSeeder extends Seeder { /** * Run the database seeds. * * @return void */ public function run() { //Added Start $products = [ [ 'name' => 'PHP', 'image' => 'https://dummyimage.com/200x200/4D588E/fff&text=PHP', 'price' => 120, 'description' => 'PHP Language' ], [ 'name' => 'Laravel', 'image' => 'https://dummyimage.com/200x200/E83A2D/fff&text=Laravel', 'price' => 220, 'description' => 'Laravel freamwork' ], [ 'name' => 'Python', 'image' => 'https://dummyimage.com/200x200/000/00ff04&text=python', 'price' => 300, 'description' => 'Python Language' ], [ 'name' => 'Codeigniter', 'image' => 'https://dummyimage.com/200x200/F03B06/000&text=CI', 'price' => 110, 'description' => 'Codeigniter freamwork' ] ]; foreach ($products as $key => $value) { Product::create($value); } //Added End } }
Now run the seeder using below command:
php artisan db:seed --class=ProductSeeder
Create Controller
Now, we need to create ProductController and copy bellow code on that file:
php artisan make:controller ProductController
<?php #app\Http\Controllers\ProductController.php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Product; //added class ProductController extends Controller { public function index() { $products = Product::all(); return view('products', compact('products')); } public function cart() { return view('cart'); } public function addToCart($id) { $product = Product::findOrFail($id); $cart = session()->get('cart', []); if(isset($cart[$id])) { $cart[$id]['quantity']++; } else { $cart[$id] = [ "name" => $product->name, "image" => $product->image, "price" => $product->price, "quantity" => 1 ]; } session()->put('cart', $cart); return redirect()->back()->with('success', 'Product add to cart successfully!'); } public function update(Request $request) { if($request->id && $request->quantity){ $cart = session()->get('cart'); $cart[$request->id]["quantity"] = $request->quantity; session()->put('cart', $cart); session()->flash('success', 'Cart successfully updated!'); } } public function remove(Request $request) { if($request->id) { $cart = session()->get('cart'); if(isset($cart[$request->id])) { unset($cart[$request->id]); session()->put('cart', $cart); } session()->flash('success', 'Product successfully removed!'); } } }
Define Route
We need to create some routes for add-to-cart functionality.
<?php #routes/web.php use Illuminate\Support\Facades\Route; use App\Http\Controllers\ProductController; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::get('/', [ProductController::class, 'index']); Route::get('cart', [ProductController::class, 'cart'])->name('cart'); Route::get('add-to-cart/{id}', [ProductController::class, 'addToCart'])->name('add_to_cart'); Route::patch('update-cart', [ProductController::class, 'update'])->name('update_cart'); Route::delete('remove-from-cart', [ProductController::class, 'remove'])->name('remove_from_cart');
Create Blade Files
Now, the Last step we need to create blade files for the layout, cart page, and products.
resources/views/layout.blade.php
<!DOCTYPE html>
<html>
<head>
<title>How to create add to cart functionality in Laravel - Devnote.in</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"></script>
<link href="{{ asset('css/styles.css') }}" rel="stylesheet">
</head>
<body>
<div class="container">
<div class="row">
<div class="col-lg-12 col-sm-12 col-12">
<div class="dropdown">
<button type="button" class="btn btn-success" data-toggle="dropdown">
<i class="fa fa-shopping-cart" aria-hidden="true"></i> Cart <span class="badge badge-pill badge-danger">{{ count((array) session('cart')) }}</span>
</button>
<div class="dropdown-menu">
<div class="row total-header-section">
@php $total = 0 @endphp
@foreach((array) session('cart') as $id => $details)
@php $total += $details['price'] * $details['quantity'] @endphp
@endforeach
<div class="col-lg-12 col-sm-12 col-12 total-section text-right">
<p>Total: <span class="text-info">$ {{ $total }}</span></p>
</div>
</div>
@if(session('cart'))
@foreach(session('cart') as $id => $details)
<div class="row cart-detail">
<div class="col-lg-4 col-sm-4 col-4 cart-detail-img">
<img src="{{ $details['image'] }}" />
</div>
<div class="col-lg-8 col-sm-8 col-8 cart-detail-product">
<p>{{ $details['name'] }}</p>
<span class="price text-info"> ${{ $details['price'] }}</span> <span class="count"> Quantity:{{ $details['quantity'] }}</span>
</div>
</div>
@endforeach
@endif
<div class="row">
<div class="col-lg-12 col-sm-12 col-12 text-center checkout">
<a href="{{ route('cart') }}" class="btn btn-primary btn-block">View all</a>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<br/>
<div class="container">
@if(session('success'))
<div class="alert alert-success">
{{ session('success') }}
</div>
@endif
@yield('content')
</div>
@yield('scripts')
</body>
</html>
resources/views/products.blade.php
@extends('layout')
@section('content')
<div class="row">
@foreach($products as $product)
<div class="col-xs-18 col-sm-6 col-md-3">
<div class="img_thumbnail">
<img src="{{ $product->image }}" alt="">
<div class="caption">
<h4>{{ $product->name }}</h4>
<p>{{ $product->description }}</p>
<p><strong>Price: </strong> {{ $product->price }}$</p>
<p class="btn-holder"><a href="{{ route('add_to_cart', $product->id) }}" class="btn btn-success btn-block text-center" role="button">Add to cart</a> </p>
</div>
</div>
</div>
@endforeach
</div>
@endsection
resources/views/cart.blade.php
@extends('layout')
@section('content')
<table id="cart" class="table table-hover table-condensed">
<thead>
<tr>
<th style="width:50%">Product</th>
<th style="width:10%">Price</th>
<th style="width:8%">Quantity</th>
<th style="width:22%" class="text-center">Subtotal</th>
<th style="width:10%"></th>
</tr>
</thead>
<tbody>
@php $total = 0 @endphp
@if(session('cart'))
@foreach(session('cart') as $id => $details)
@php $total += $details['price'] * $details['quantity'] @endphp
<tr data-id="{{ $id }}">
<td data-th="Product">
<div class="row">
<div class="col-sm-3 hidden-xs"><img src="{{ $details['image'] }}" width="100" height="100" class="img-responsive"/></div>
<div class="col-sm-9">
<h4 class="nomargin">{{ $details['name'] }}</h4>
</div>
</div>
</td>
<td data-th="Price">${{ $details['price'] }}</td>
<td data-th="Quantity">
<input type="number" value="{{ $details['quantity'] }}" class="form-control quantity cart_update" min="1" />
</td>
<td data-th="Subtotal" class="text-center">${{ $details['price'] * $details['quantity'] }}</td>
<td class="actions" data-th="">
<button class="btn btn-danger btn-sm cart_remove"><i class="fa fa-trash-o"></i> Delete</button>
</td>
</tr>
@endforeach
@endif
</tbody>
<tfoot>
<tr>
<td colspan="5" class="text-right"><h3><strong>Total ${{ $total }}</strong></h3></td>
</tr>
<tr>
<td colspan="5" class="text-right">
<a href="{{ url('/') }}" class="btn btn-danger"> <i class="fa fa-arrow-left"></i> Continue Shopping</a>
<button class="btn btn-success"><i class="fa fa-money"></i> Checkout</button>
</td>
</tr>
</tfoot>
</table>
@endsection
@section('scripts')
<script type="text/javascript">
$(".cart_update").change(function (e) {
e.preventDefault();
var ele = $(this);
$.ajax({
url: '{{ route('update_cart') }}',
method: "patch",
data: {
_token: '{{ csrf_token() }}',
id: ele.parents("tr").attr("data-id"),
quantity: ele.parents("tr").find(".quantity").val()
},
success: function (response) {
window.location.reload();
}
});
});
$(".cart_remove").click(function (e) {
e.preventDefault();
var ele = $(this);
if(confirm("Do you really want to remove?")) {
$.ajax({
url: '{{ route('remove_from_cart') }}',
method: "DELETE",
data: {
_token: '{{ csrf_token() }}',
id: ele.parents("tr").attr("data-id")
},
success: function (response) {
window.location.reload();
}
});
}
});
</script>
@endsection
public/css/styles.css
.img_thumbnail {
position: relative;
padding: 0px;
margin-bottom: 20px;
}
.img_thumbnail img {
width: 100%;
}
.img_thumbnail .caption{
margin: 7px;
text-align: center;
}
.dropdown{
float:right;
padding-right: 30px;
}
.btn{
border:0px;
margin:10px 0px;
box-shadow:none !important;
}
.dropdown .dropdown-menu{
padding:20px;
top:30px !important;
width:350px !important;
left:-110px !important;
box-shadow:0px 5px 30px black;
}
.total-header-section{
border-bottom:1px solid #d2d2d2;
}
.total-section p{
margin-bottom:20px;
}
.cart-detail{
padding:15px 0px;
}
.cart-detail-img img{
width:100%;
height:100%;
padding-left:15px;
}
.cart-detail-product p{
margin:0px;
color:#000;
font-weight:500;
}
.cart-detail .price{
font-size:12px;
margin-right:10px;
font-weight:500;
}
.cart-detail .count{
color:#000;
}
.checkout{
border-top:1px solid #d2d2d2;
padding-top: 15px;
}
.checkout .btn-primary{
border-radius:50px;
}
.dropdown-menu:before{
content: " ";
position:absolute;
top:-20px;
right:50px;
border:10px solid transparent;
border-bottom-color:#fff;
}
Now run our example so run below command:
php artisan serve
Output
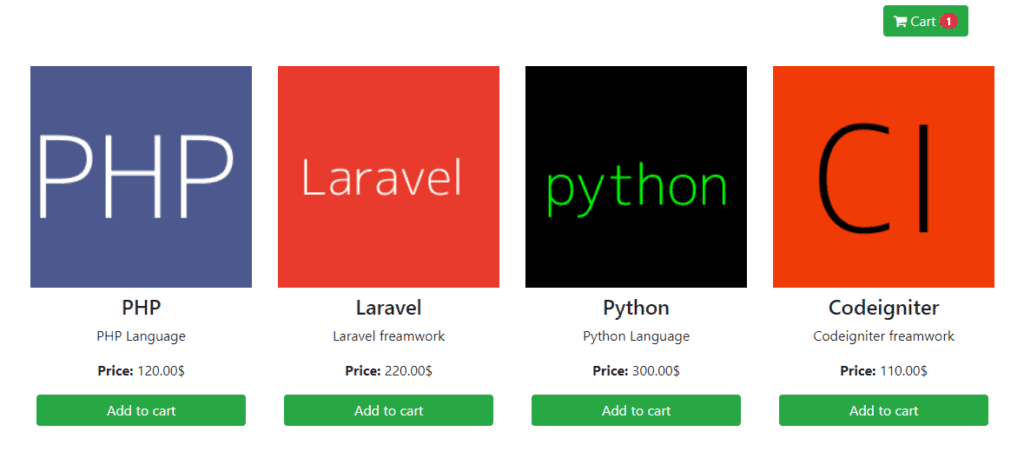
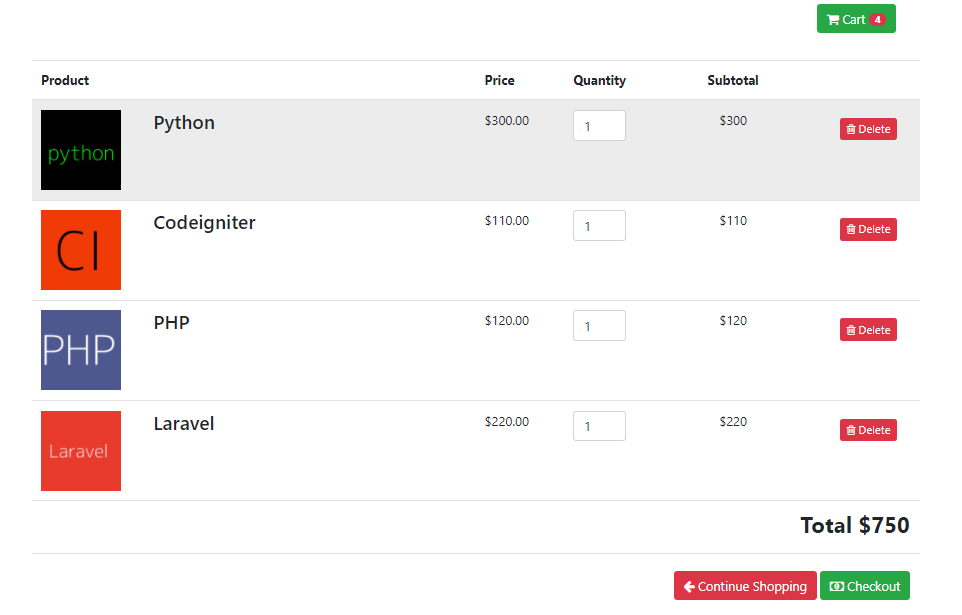
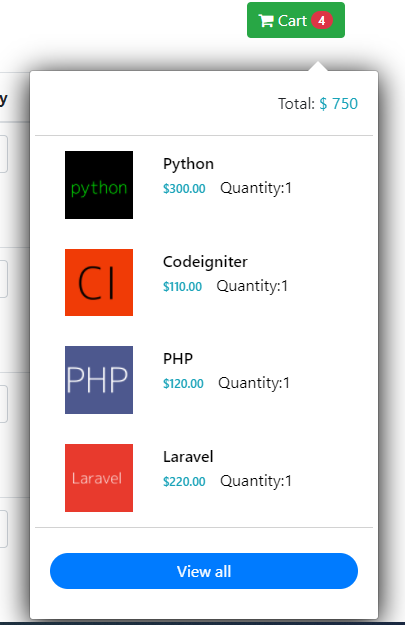