How to read RSS feeds using PHP
In this tutorial, we will learn How to read RSS feeds using PHP. you can use PHP to read RSS feeds of the websites and show recent post lists. RSS (Rich Site Summary) feed uses people who want to receive the latest posts updates from their favorite websites.
We will use PHP default provides simplexml_load_file() function for reading data from XML file. We are reading RSS feeds bypassing the feed URL to this function. we display all detail of the article title, links, and description using for each loop.
Example: PHP file to display the RSS Feed
We create a PHP file and save it with a name feed.php
<html>
<head>
<title>How to read RSS feeds using PHP</title>
<style>
body {
width:100%;
margin:0 auto;
padding:0px;
font-family:helvetica;
background-color:#A5D1F5;
}
#main_wrapper {
text-align:center;
margin:0 auto;
padding:0px;
width:995px;
}
#main_wrapper h1 {
margin-top:100px;
font-size:40px;
}
#main_wrapper h1 p {
font-size:17px;
}
#main_wrapper #feeds {
background-color:white;
width:550px;
height:auto;
overflow-y:scroll;
margin: 0 auto;
margin-top:20px;
text-align:left;
border:1px solid red;
padding:10px;
}
#main_wrapper #feeds h2 {
font-size:17px;
}
#main_wrapper #feeds .title a {
text-decoration:none;
color:#0010FF;
}
</style>
</head>
<body>
<div id="main_wrapper">
<div id="feeds">
<?php
$rss = simplexml_load_file('https://devnote.in/feeds/');
echo '<h3>'. $rss->channel->title . '</h3>';
foreach ($rss->channel->item as $item) {
echo '<p class="title"><a href="'. $item->link .'">' . $item->title . "</a></p>";
echo "<p class='desc'>" . substr($item->description, 0, 300) . "</p>";
}
?>
</div>
</div>
</body>
</html>
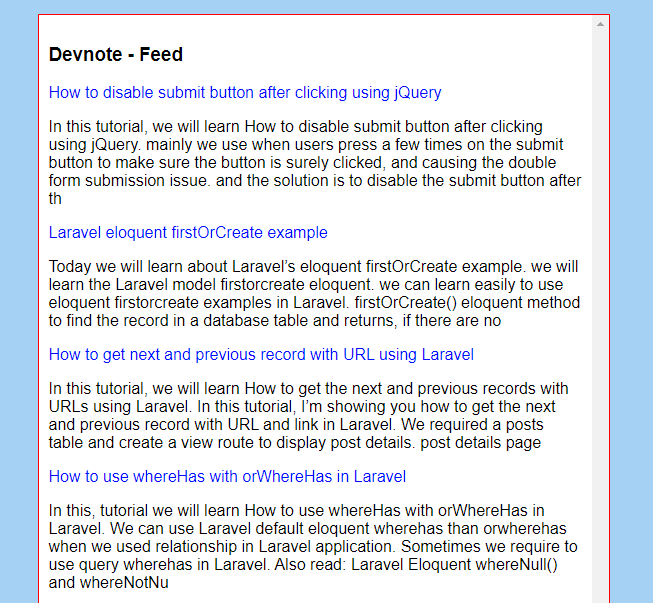
Example: read RSS feeds using PHP
<html>
<head>
<title>How to read RSS feeds using PHP</title>
<style>
body{
width: 410px;
margin: 0 auto;
}
.table {
width: 100%;
border-spacing: initial;
margin: 20px 0px;
word-break: break-word;
table-layout: auto;
line-height:2.1em;
color:#434;
}
.table th {
background: #000;
padding: 8px 30px 5px;
text-align: left;
color:#FFF;
}
.table td {
border-bottom: #e2e2e2 1px solid;
background-color: #FFF;
padding: 5px;
}
.table td a.title{
text-decoration: none;
color:#FFF;
font-weight: 600;
}
</style>
</head>
<body>
<?php $rss = simplexml_load_file('https://devnote.in/feeds/'); ?>
<table class="table">
<tbody>
<tr><th><strong>How to read RSS feeds using PHP</strong></th></tr>
<?php
if(!empty($rss)) {
$index = 0;
foreach ($rss->channel->item as $values) {
if($index >= 10) { break; } ?>
<tr>
<td valign="top">
<div>
<a class="title" href="<?php echo $values->link; ?>">
<?php echo $values->title; ?>
</a>
</div>
<div>
<?php echo implode(' ', array_slice(explode(' ', $values->description), 0, 23)) . "..."; ?>
</div>
</td>
</tr>
<?php
$index++;
}
}
?>
</tbody>
</table>
</body>
</html>
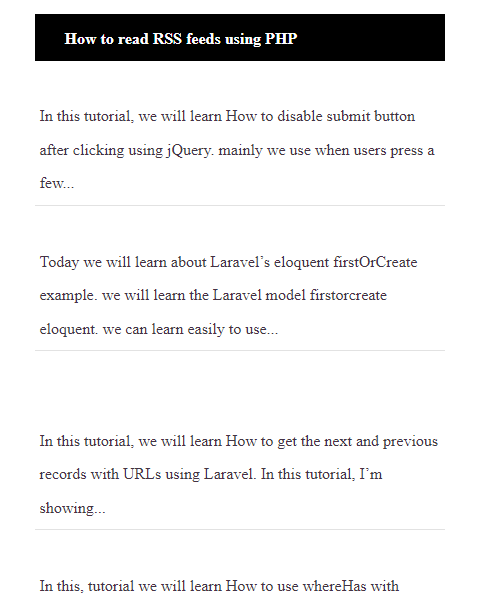