Laravel 9 Ajax Example
In this tutorial, we will learn Laravel 9 Ajax Example. this example will help you with the Laravel 9 jquery ajax post example. This article will give you a simple example of jquery ajax in Laravel 9. you will learn Laravel 9 ajax from submitting examples.
Ajax requirement of any PHP project, and we are always without page refresh data should store in the database, retrieve data from the database. And it is possible only with jquery ajax. if you need to write an ajax form to submit data in Laravel 9 then I will show you how we can pass data with an ajax request and get on the controller in Laravel. so just follow step-by-step and you will learn how to use ajax requests in your Laravel 9.
Step 1: Create Controller
First, We create a Controller, and We will add two new methods. The first is handle view layout and the second is post ajax request.
<?php
#app/Http/Controllers/ProductsController.php
namespace App\Http\Controllers;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use Log;
class ProductsController extends Controller
{
public function create() {
return view('product.create');
}
public function store(Request $request) {
$input = $request->all();
Log::info($input);
return response()->json(['success'=>'Data retrive from request successfully.']);
}
}
Step 2: Create Routes
Now, we add two routes in the web.php file inside routes
folder, and these two routes are used for displaying view and another post ajax.
<?php
#routes/web.php
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/', function() {
return view('welcome');
});
Route::get('product-create', 'App\Http\Controllers\ProductsController@create');
Route::post('product-store', 'App\Http\Controllers\ProductsController@store')->name('product.store');
Step 3: Create Blade File
Finally, we require to create a product/create.blade.php
file and here we will write the below code with jquery ajax and pass the Laravel token. And blade file is very important in ajax requests.
<!DOCTYPE html>
<html>
<head>
<title>Laravel 9 Ajax Example</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@3.3.7/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script>
<meta name="csrf-token" content="{{ csrf_token() }}" />
</head>
<body>
<div class="container">
<h1>Laravel 9 Ajax Example</h1>
<div id="message_display"></div>
<form id="products">
<div class="form-group">
<label>Product name:</label>
<input type="text" name="product_name" id="product_name" class="form-control" placeholder="Product Name" required>
</div>
<div class="form-group">
<label>Product Description:</label>
<textarea name="product_description" id="product_description" placeholder="Product Description" class="form-control"></textarea>
</div>
<div class="form-group">
<button class="btn btn-success btn-submit add_product">Submit</button>
</div>
</form>
</div>
</body>
<script type="text/javascript">
$.ajaxSetup({
headers: {
'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content')
}
});
$(".add_product").click(function(e) {
e.preventDefault();
var product_name = $("#product_name").val();
var product_description = $("#product_description").val();
if(product_name == '' || product_description == '') {
$('#message_display').html('<div class="alert alert-danger">Please enter proper data.</div>');
$('#message_display div').delay(1000).hide(0);
return false;
}
$.ajax({
type: 'POST',
url: "{{ route('product.store') }}",
data: {
product_name: product_name,
product_description: product_description
},
success: function(data) {
$('#message_display').html('<div class="alert alert-success">' + data.success + '</div>');
$('#message_display div').delay(2000).hide(0);
$('#products')[0].reset();
}
});
});
</script>
</html>
Now run your example and see the below Output.
Step 4: Output
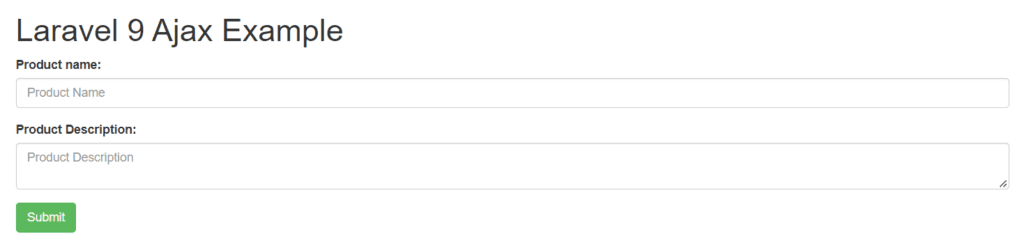
#storage\logs\laravel.log
[2022-09-15 07:50:26] local.INFO: array (
'product_name' => 'Iphone 14 Pro',
'product_description' => 'Lorem ipsum dolor sit amet consectetur adipisicing elit. Esse architecto, laudantium incidunt quos voluptatum vitae obcaecati assumenda numquam ab aliquid eligendi sint, doloribus quo eius eos ea, modi ad animi!',
)