How to create a Livewire Form in Laravel
Today we will create How to create a Livewire Form in Laravel. I will give a very simple example of laravel livewire Form tutorial. When the user implements a laravel livewire form, he gets some errors. So In this tutorial, I will give a simple example of How to install Livewire Form. I will give you a very simple example of creating a Form with a contacts table.
Also read: How to Upload Profile in Registration Form in Laravel?
Also, You can use laravel livewire form with laravel 6, laravel 7, laravel 8 and laravel 9 versions.
In this tutorial, I will give you a simple example of creating a contact form with a name and email and I will store that data in the database without refreshing the page. we will use only livewire/livewire
the package.
How to create a Livewire Form in Laravel
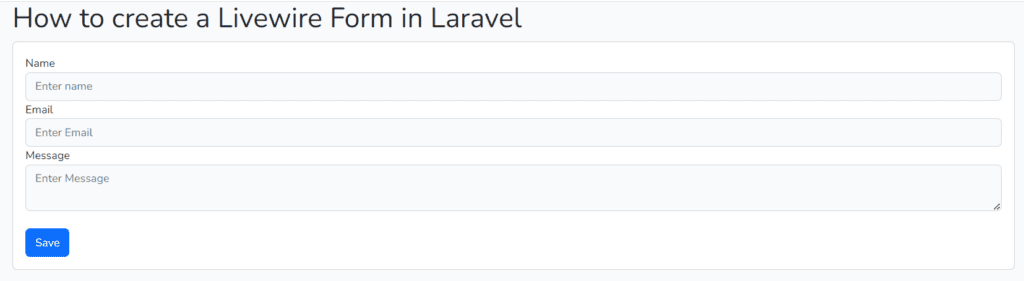
Table of concept
- Install Livewire
- Create Migration and Model
- Create Component
- Create a Route
- Create a View File
1. Install Livewire
Also read: How to create Livewire Pagination in Laravel step 1.
2. Create Migration and Model
In this step, We can create a database migration for the contacts table and also we will create a model for the contacts table. So let’s start:
php artisan make:model Contact -m

Migration File
<?php
#database\migrations/2023_02_28_050939_create_contacts_table.php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('contacts', function (Blueprint $table) {
$table->id();
$table->string('name'); //Added
$table->string('email'); //Added
$table->text('message'); //Added
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('contacts');
}
};
And run the below command:
php artisan migrate
Model File
<?php
#app/Models/Contact.php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Contact extends Model
{
use HasFactory;
/**
* Write code on Method
*
* @return response()
*/
protected $fillable = [
'name', 'email', 'message' // Added
];
}
3. Create Component
Now, we will create a livewire component using the below command.
Also read: How to create Livewire Datatables Using Laravel
php artisan make:livewire contact-form
The above command creates a contact form component. and they created two files:
CLASS: app/Http/Livewire//ContactForm.php
VIEW: E:\xampp\htdocs\practise\resources\views/livewire/contact-form.blade.php
Now both file we will update both as the below code.
<?php
#app/Http/Livewire//ContactForm.php
namespace App\Http\Livewire;
use Livewire\Component;
use App\Models\Contact; //Added
class ContactForm extends Component
{
/* Added Start */
public $name;
public $email;
public $message;
public function submit()
{
$validatedData = $this->validate([
'name' => 'required',
'email' => 'required|email',
'message' => 'required',
]);
Contact::create($validatedData);
return redirect()->to('/contact-us');
}
/* Added End */
public function render()
{
return view('livewire.contact-form');
}
}
resources/views/livewire/contact-form.blade.php
<form wire:submit.prevent="submit">
<div class="form-group">
<label for="name">Name</label>
<input type="text" class="form-control" id="name" placeholder="Enter name" wire:model="name">
@error('name') <span class="text-danger">{{ $message }}</span> @enderror
</div>
<div class="form-group">
<label for="email">Email</label>
<input type="text" class="form-control" id="email" placeholder="Enter Email" wire:model="email">
@error('email') <span class="text-danger">{{ $message }}</span> @enderror
</div>
<div class="form-group">
<label for="message">Message</label>
<textarea class="form-control" id="message" placeholder="Enter Message" wire:model="message"></textarea>
@error('Message') <span class="text-danger">{{ $message }}</span> @enderror
</div>
<br>
<button type="submit" class="btn btn-primary">Save</button>
</form>
4. Create a Route
Now we will create one route for calling our example.
#routes/web.php
Route::get('contact-us', function () {
return view('contact.index');
});
5. Create a View File
Last, we will create a blade file for the call form route. in this file, we will use @livewireStyles and @livewireScripts.
resources/views/contact/index.blade.php
<!DOCTYPE html>
<html>
<head>
<title>How to create a Livewire Form in Laravel</title>
@vite(['resources/sass/app.scss', 'resources/js/app.js'])
</head>
<body>
<div class="container">
<h1>How to create a Livewire Form in Laravel</h1>
<div class="card">
<div class="card-body">
@livewire('contact-form')
</div>
</div>
</div>
</body>
@livewireScripts
</html>