How to create Analog Clock in HTML CSS & JavaScript
Today we will learn How to create Analog Clock in HTML CSS & JavaScript. In this example we will create an analog clock using HTML, CSS, and JavaScript is an excellent way to improve your coding skills in web designing and development. I will provide guidance on how to create dark and light mode options for the clock’s functionality.
How to create Analog Clock in HTML CSS & JavaScript
Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>How to create Analog Clock in HTML CSS & JavaScript</title>
<style>
@import url('https://fonts.googleapis.com/css2?family=Montserrat&family=Roboto:wght@100;300;400;500;700;900&display=swap');
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Montserrat', sans-serif;
font-family: 'Roboto', sans-serif;
}
:root {
--primary-color: #f6f7fb;
--white-color: #fff;
--black-color: #18191a;
--red-color: #ff1900;
}
body {
display: flex;
min-height: 100vh;
align-items: center;
justify-content: center;
background: var(--primary-color);
}
body.dark {
--primary-color: #242526;
--white-color: #18191a;
--black-color: #fff;
--red-color: #ff1900;
}
.container {
display: flex;
flex-direction: column;
align-items: center;
gap: 60px;
}
.container .clocks {
display: flex;
height: 400px;
width: 400px;
border-radius: 50%;
align-items: center;
justify-content: center;
background: var(--white-color);
box-shadow: 0 15px 25px rgba(0, 0, 0, 0.1), 0 25px 45px rgba(0, 0, 0,
0.1);
position: relative;
}
.clocks label {
position: absolute;
inset: 20px;
text-align: center;
transform: rotate(calc(var(--i) * (360deg / 12)));
}
.clocks label span {
display: inline-block;
font-size: 30px;
font-weight: 600;
color: var(--black-color);
transform: rotate(calc(var(--i) * (-360deg / 12)));
}
.container .indicators {
position: absolute;
height: 10px;
width: 10px;
display: flex;
justify-content: center;
}
.indicators::before {
content: "";
position: absolute;
height: 100%;
width: 100%;
border-radius: 50%;
z-index: 100;
background: var(--black-color);
border: 4px solid var(--red-color);
}
.indicators .hand {
position: absolute;
height: 130px;
width: 4px;
bottom: 0;
border-radius: 25px;
transform-origin: bottom;
background: var(--red-color);
}
.hand.minutes {
height: 120px;
width: 5px;
background: var(--black-color);
}
.hand.hours {
height: 100px;
width: 8px;
background: var(--black-color);
}
.switch-mode {
padding: 10px 20px;
border-radius: 8px;
font-size: 22px;
font-weight: 400;
display: inline-block;
color: var(--white-color);
background: var(--black-color);
box-shadow: 0 5px 10px rgba(0, 0, 0, 0.1);
cursor: pointer;
}
</style>
</head>
<body>
<div class="container">
<div class="clocks">
<label style="--i: 1"><span>1</span></label>
<label style="--i: 2"><span>2</span></label>
<label style="--i: 3"><span>3</span></label>
<label style="--i: 4"><span>4</span></label>
<label style="--i: 5"><span>5</span></label>
<label style="--i: 6"><span>6</span></label>
<label style="--i: 7"><span>7</span></label>
<label style="--i: 8"><span>8</span></label>
<label style="--i: 9"><span>9</span></label>
<label style="--i: 10"><span>10</span></label>
<label style="--i: 11"><span>11</span></label>
<label style="--i: 12"><span>12</span></label>
<div class="indicators">
<span class="hand hours"></span>
<span class="hand minutes"></span>
<span class="hand seconds"></span>
</div>
</div>
<div class="switch-mode">Dark Mode</div>
</div>
<script>
const body = document.querySelector("body"),
hours = document.querySelector(".hours"),
minutes = document.querySelector(".minutes"),
seconds = document.querySelector(".seconds"),
modeSwitch = document.querySelector(".switch-mode");
if (localStorage.getItem("mode") === "Dark Mode") {
body.classList.add("dark");
modeSwitch.textContent = "Light Mode";
}
modeSwitch.addEventListener("click", () => {
body.classList.toggle("dark");
const isDarkMode = body.classList.contains("dark");
modeSwitch.textContent = isDarkMode ? "Light Mode" : "Dark Mode";
localStorage.setItem("mode", isDarkMode ? "Dark Mode" : "Light Mode");
});
const everySecondTimeUpdate = () => {
let date = new Date(),
secDeg = (date.getSeconds() / 60) * 360,
minDeg = (date.getMinutes() / 60) * 360,
hrDeg = (date.getHours() / 12) * 360;
seconds.style.transform = `rotate(${secDeg}deg)`;
minutes.style.transform = `rotate(${minDeg}deg)`;
hours.style.transform = `rotate(${hrDeg}deg)`;
};
setInterval(everySecondTimeUpdate, 1000);
everySecondTimeUpdate();
</script>
</body>
</html>
Output
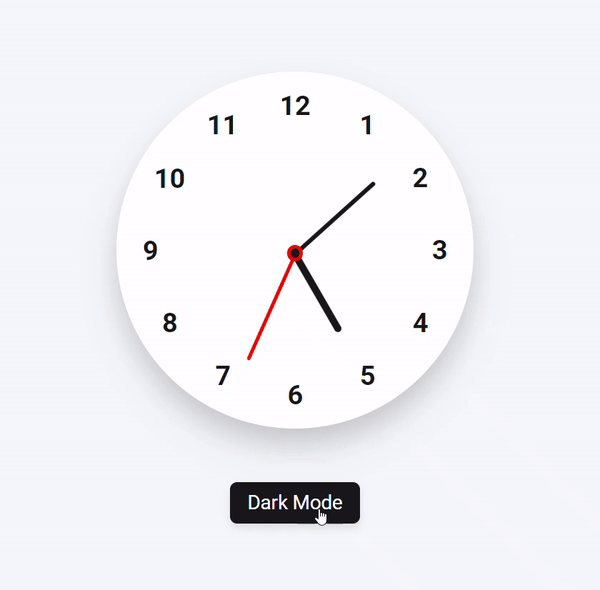