How to Implement Token-based authentication using Django
Django is the most popular web development framework. the most popular python package is Django to develop rest API and it made is really easier from authentication. the authentication which is used today :
Create django-project
Also read : How to Install Django | Basic Configuration
Now make a project inside the folder.
$ django-admin startproject rest_api
A rest_api folder is created having a manage.py file and rest_api folder containing settings.py which containing all the settings of your project.
Also read : Django Invalid HTTP_HOST Header | ALLOWED_HOSTS
Django rest framework Installing
$ pip install djangorestframework
Now update the settings.py file. following step:
settings.py
#rest_api/settings.py INSTALLED_APPS = [ ... 'rest_framework', #added 'rest_framework.authtoken', #added ] #added REST_FRAMEWORK = { 'DEFAULT_AUTHENTICATION_CLASSES': ( 'rest_framework.authentication.TokenAuthentication', ), 'DEFAULT_PERMISSION_CLASSES': ( 'rest_framework.permissions.IsAuthenticated', ) }
Login function and api route
We write the login function. it returns the token with the user detail and makes the API route for the same use.
views.py
#rest_api/views.py
from rest_framework.decorators import api_view, permission_classes
from django.views.decorators.csrf import csrf_exempt
from rest_framework.authtoken.models import Token
from rest_framework.permissions import AllowAny
from django.contrib.auth import authenticate
from rest_framework.status import (
HTTP_400_BAD_REQUEST,
HTTP_404_NOT_FOUND,
HTTP_200_OK
)
from rest_framework.response import Response
@csrf_exempt
@api_view(["POST"])
@permission_classes((AllowAny,))
def login(request):
username = request.data.get("username")
password = request.data.get("password")
if username is None or password is None:
return Response({'error': 'Please enter username & password!'},status=HTTP_400_BAD_REQUEST)
user = authenticate(username=username, password=password)
if not user:
return Response({'error': 'Please enter Valid Creadentials!'},status=HTTP_404_NOT_FOUND)
token, _ = Token.objects.get_or_create(user=user)
return Response({'token': token.key}, status=HTTP_200_OK)
Now make API route in the urls.py file.
urls.py
#rest_api/urls.py
from django.contrib import admin
from django.urls import path
from . import views
urlpatterns = [
path('admin/', admin.site.urls),
path('api/login', views.login)
]
Now, it time for testing the login api. But before that run migrations.
$ python manage.py makemigrations
$ python manage.py migrate
Testing the Login api route
The login API route we have to make a user, you can make use either with the admin panel or using the command line.
$ python manage.py createsuperuser
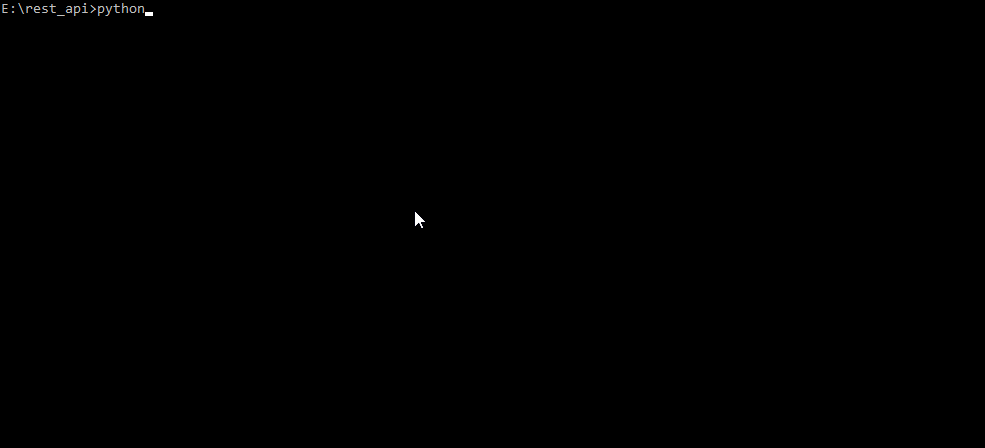
Now i am test the api in the postman :
url : http://127.0.0.1:8080/api/login
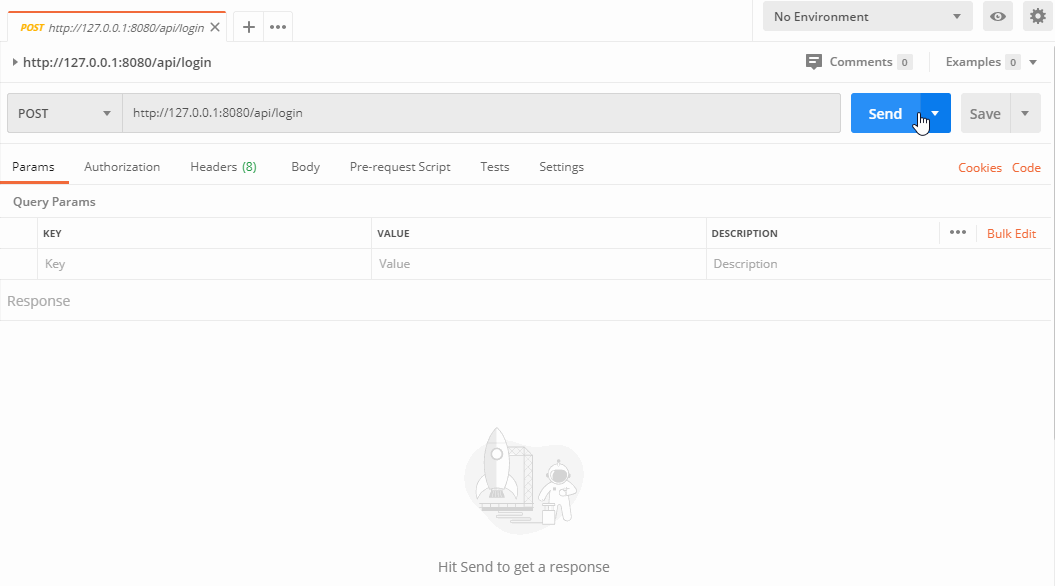
A devnote API that is authenticated and then tries to access the API using the token.
The following things in the views.py file.
views.py
#rest_api/views.py
@csrf_exempt
@api_view(["GET"])
def devnote_api(request):
data = "How to Implement Token-based authentication using Django"
return Response({'data': data}, status=HTTP_200_OK)
Add your route in the urls.py.
urls.py
#rest_api/urls.py
from django.contrib import admin
from django.urls import path
from . import views
urlpatterns = [
path('admin/', admin.site.urls),
path('api/login', views.login),
path('api/devnote', views.devnote_api) #added
]
Token access authenticated
Now we try to access the devnote api without or with the token and see the response.
Without token
url : http://127.0.0.1:8080/api/devnote
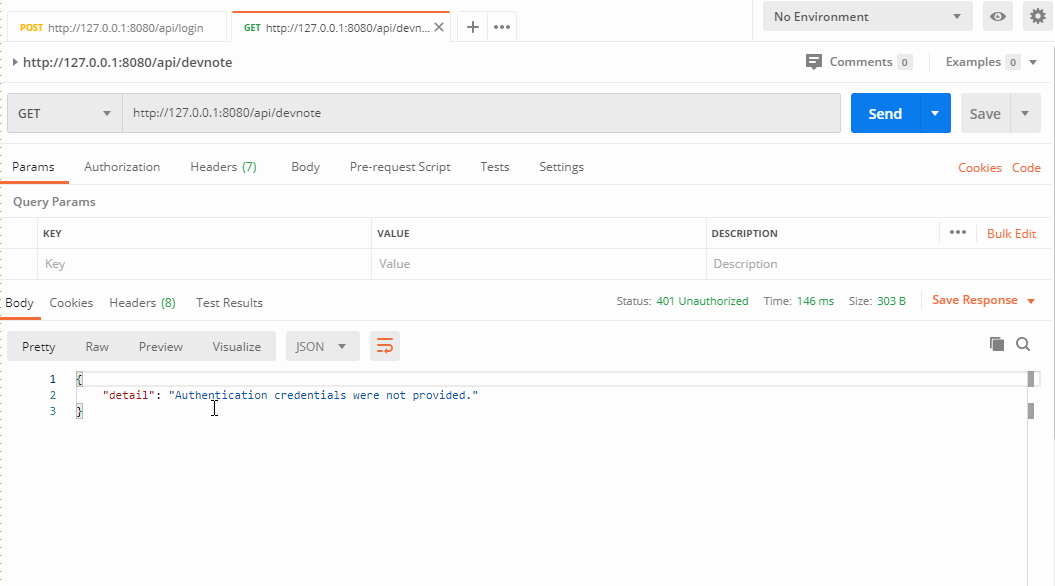
url : http://127.0.0.1:8080/api/devnote
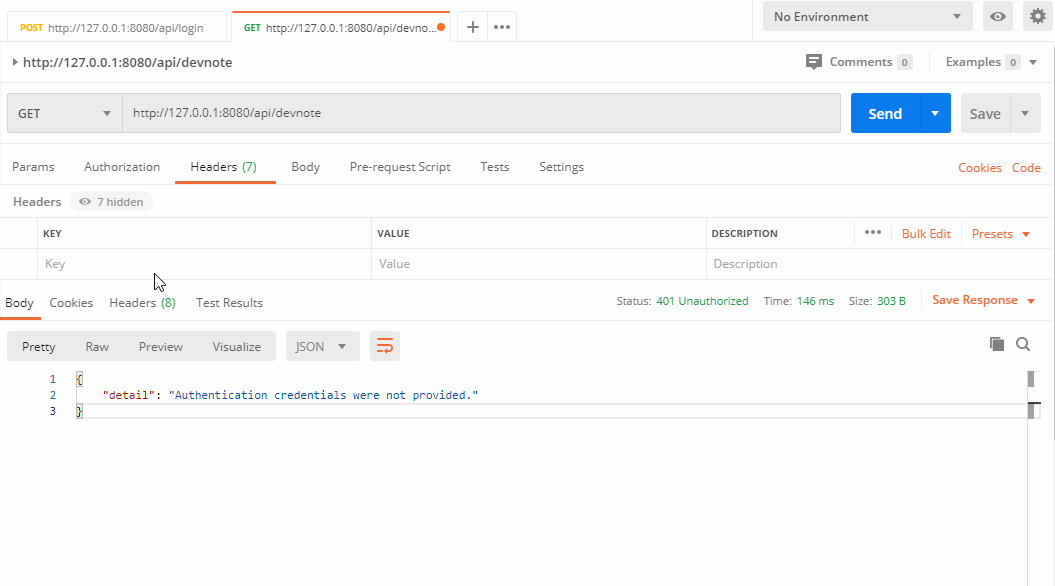