Change Password Functionality using Laravel 8
In this tutorial, we will discuss Change Password Functionality using Laravel 8. Change passwords mostly use carries a lot of sensitive and important data, so keeping work data safe is a major priority. Laravel Authentication default provided Change password functionality.
Change Password Form Page
First, we will create a change password form and the required route and controller method for the same.
Create View File
Now, create our change password view file named change-password.blade.php
.
resources/views/auth/passwords/change-password.blade.php
@extends('layouts.app')
@section('content')
<div class="container">
<div class="row">
<div class="col-md-10 offset-2">
<div class="panel panel-default">
<h2>Change password</h2>
<div class="panel-body">
@if (session('error'))
<div class="alert alert-danger">
{{ session('error') }}
</div>
@endif
@if (session('success'))
<div class="alert alert-success">
{{ session('success') }}
</div>
@endif
@if($errors)
@foreach ($errors->all() as $error)
<div class="alert alert-danger">{{ $error }}</div>
@endforeach
@endif
<form class="form-horizontal" method="POST" action="{{ route('changePasswordPost') }}">
{{ csrf_field() }}
<div class="form-group{{ $errors->has('current-password') ? ' has-error' : '' }}">
<label for="new-password" class="col-md-4 control-label">Current Password</label>
<div class="col-md-6">
<input id="current-password" type="password" class="form-control" name="current-password" required>
@if ($errors->has('current-password'))
<span class="help-block">
<strong>{{ $errors->first('current-password') }}</strong>
</span>
@endif
</div>
</div>
<div class="form-group{{ $errors->has('new-password') ? ' has-error' : '' }}">
<label for="new-password" class="col-md-4 control-label">New Password</label>
<div class="col-md-6">
<input id="new-password" type="password" class="form-control" name="new-password" required>
@if ($errors->has('new-password'))
<span class="help-block">
<strong>{{ $errors->first('new-password') }}</strong>
</span>
@endif
</div>
</div>
<div class="form-group">
<label for="new-password-confirm" class="col-md-4 control-label">Confirm New Password</label>
<div class="col-md-6">
<input id="new-password-confirm" type="password" class="form-control" name="new-password_confirmation" required>
</div>
</div>
<div class="form-group">
<div class="col-md-6 col-md-offset-4">
<button type="submit" class="btn btn-primary">
Change Password
</button>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
@endsection
Now, the user is logged in, and if you go to devnote.in/change-password then you should display the below page.
Create Controller
I create new HomeController
and add the supporting controller method show_change_password_form
in Controller. But you are free to put it in any other controller or create a separate controller for the change-password functionality. And we can make sure that only authenticated users can access the change password functionality.
Post Change Password Request
- We check the following things.
- The current password and the new password should not be same.
- Current password provided by user it should match in the database. And we check this by
- using
Hash::check
method. - New password Validate requirements, new password and confirm password should be same.
- Change the password for the user account and redirect back with the success message.
<?php #app\Http\Controllers\HomeController.php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; use Hash; use Auth; class HomeController extends Controller { public function showChangePasswordGet() { return view('auth.passwords.change-password'); } public function changePasswordPost(Request $request) { if (!(Hash::check($request->get('current-password'), Auth::user()->password))) { // The passwords matches return redirect()->back()->with("error","Your current password does not matches with the password."); } if(strcmp($request->get('current-password'), $request->get('new-password')) == 0){ // Current password and new password same return redirect()->back()->with("error","New Password cannot be same as your current password."); } $validatedData = $request->validate([ 'current-password' => 'required', 'new-password' => 'required|string|min:8|confirmed', ]); //Change Password $user = Auth::user(); $user->password = bcrypt($request->get('new-password')); $user->save(); return redirect()->back()->with("success","Password successfully changed!"); } }
Add routes
Add the below code into your route file.
#routes/web.php Route::get('/changePassword', [App\Http\Controllers\HomeController::class, 'showChangePasswordGet'])->name('changePasswordGet'); Route::post('/changePassword', [App\Http\Controllers\HomeController::class, 'changePasswordPost'])->name('changePasswordPost'); ===== OR Authentication check ===== Route::group(['middleware' => 'auth'], function() { Route::get('/changePassword',[App\Http\Controllers\HomeController::class, 'showChangePasswordGet'])->name('changePasswordGet'); Route::post('/changePassword',[App\Http\Controllers\HomeController::class, 'changePasswordPost'])->name('changePasswordPost'); });
Add link
We are looking to include the change Password link in the username top corner right side in the navigation bar. Include the below snippet in your resources/views/layouts/app.blade.php
file:
#resources/views/layouts/app.blade.php
<div class="dropdown-menu dropdown-menu-right" aria-labelledby="navbarDropdown">
<a class="dropdown-item" href="{{ route('logout') }}"
onclick="event.preventDefault();
document.getElementById('logout-form').submit();">
{{ __('Logout') }}
</a>
<!-- Change password -->
<a class="dropdown-item" href="{{ route('changePasswordGet') }}">Change Password </a>
<form id="logout-form" action="{{ route('logout') }}" method="POST" class="d-none">
@csrf
</form>
</div>
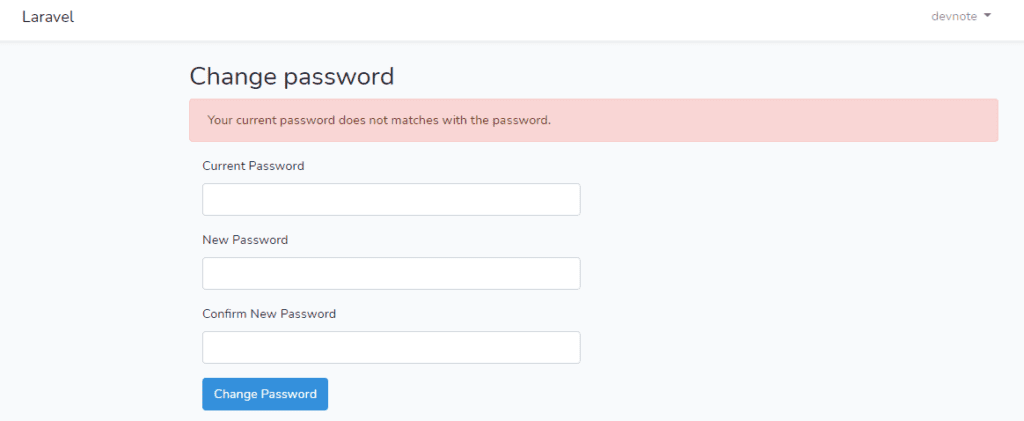
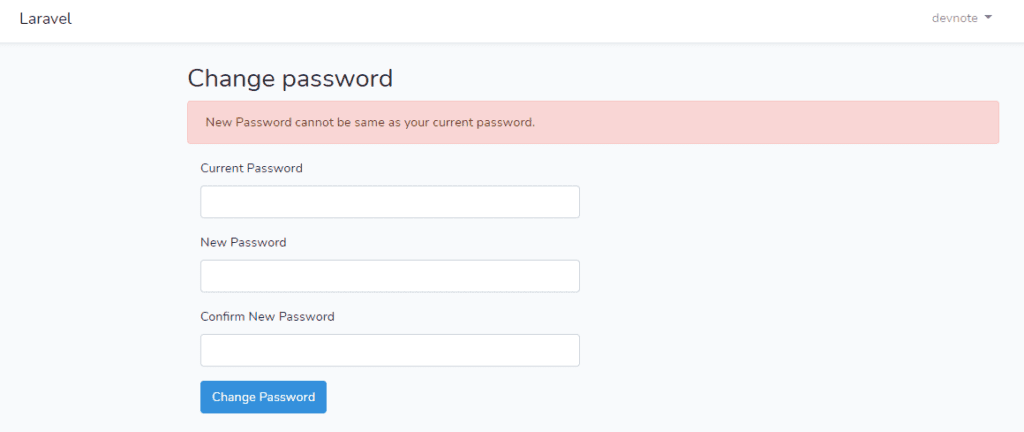
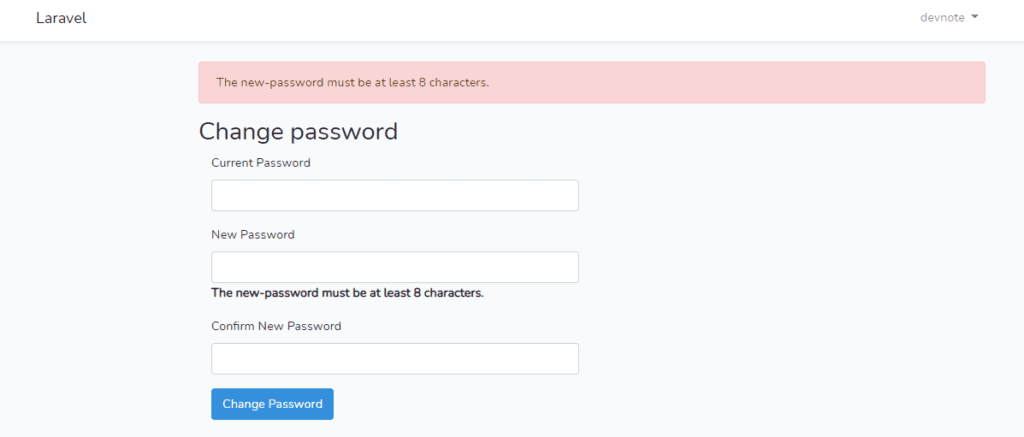
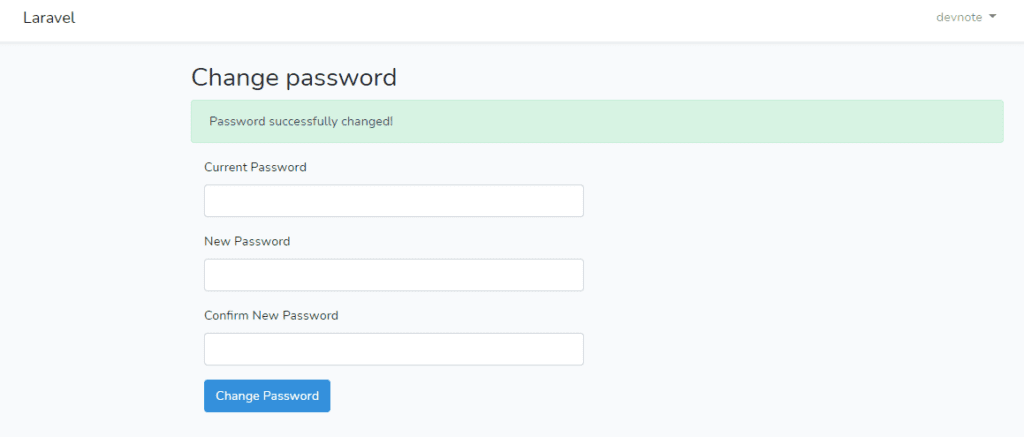