How to add Custom Fields User Profile Page in WordPress
In this tutorial, we will learn How to add Custom Fields User Profile Page in WordPress. we will add custom fields to the user profile edit page in WordPress on the admin side. WordPress Custom Fields User Profile Page is used to add some extra information for the users in his/her website. Also, we know, WordPress default provides hooks for using other features/functionality, so we can use hooks to update or manipulate according to our needs. In this post, we use some hooks to fulfill our post purpose.
If you are looking for all the below question answers? if yes. then you are right place here.
Also read: How to create wp_editor Instance in WordPress
Does the user profile page add fields in WordPress?
How to add a user profile page in the custom field?
Add Custom Fields User Profile Page in WordPress?
User profile page add custom fields using WordPress?
<?php
/**
* @param $user
* @author Devnote
*/
add_action('edit_user_profile', 'dev_custom_user_profile_fields');
add_action('show_user_profile', 'dev_custom_user_profile_fields');
function dev_custom_user_profile_fields($user) {
echo '<h2 class="custom-heading">Custom Fields:</h2>'; ?>
<table class="form-table">
<tr>
<th><label for="title">Meta Title</label></th>
<td><input type="text" name="meta_title" class="input-text form-control" id="title"/></td>
</tr>
</table>
<?php
}
?>
Above code, we add action to default hook edit_user_profile and create one input field in a callback function. ‘dev_custom_user_profile_fields’ function will display field when admin editing other user profiles. And we show the meta_title field for all profiles, then we use the show_user_profile WordPress default hook and add an action for the same callback function.
Now check custom field will display on users’ profile edit page, like the below screenshot:
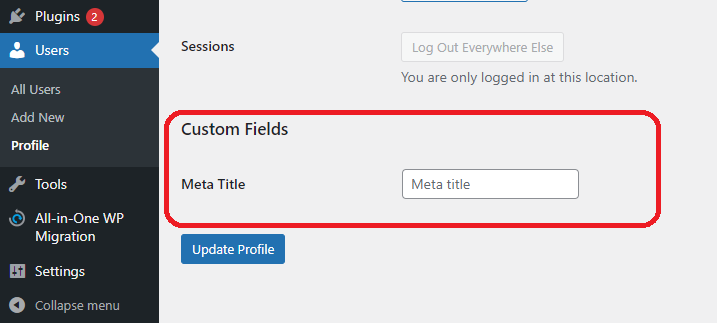
Now, we save the data for creating our custom fields. we will use edit_user_profile_update and personal_options_update WordPress default hook.
<?php
/**
* @param User Id $user_id
*/
add_action('edit_user_profile_update', 'dev_save_custom_fields_user_profile');
add_action('personal_options_update', 'dev_save_custom_fields_user_profile');
function dev_save_custom_fields_user_profile($user_id) {
$meta_title = $_POST['meta_title'];
update_user_meta($user_id, 'meta_title', $meta_title);
}
?>
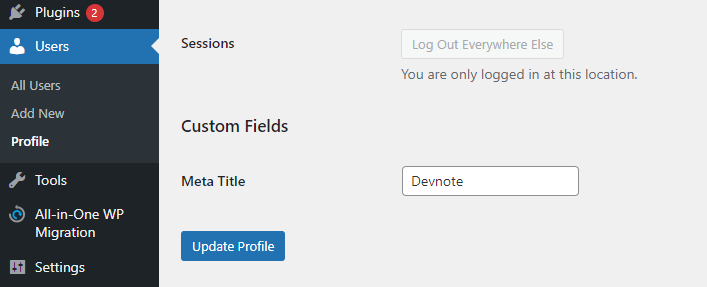
Full Code
<?php #functions.php /** * @param $user * @author Devnote */ add_action('edit_user_profile', 'dev_custom_user_profile_fields'); add_action('show_user_profile', 'dev_custom_user_profile_fields'); function dev_custom_user_profile_fields($user) { $user_data = get_user_meta($user->id); $title = isset($user_data['meta_title'][0]) ? $user_data['meta_title'][0] : ""; echo '<h2 class="custom-heading">Custom Fields</h2>'; ?> <table class="form-table"> <tr> <th><label for="title">Meta Title</label></th> <td><input type="text" name="meta_title" class="input-text form-control" value="<?php echo $title; ?>" id="title" placeholder="Meta title"/></td> </tr> </table> <?php } /** * @param User Id $user_id */ add_action('edit_user_profile_update', 'dev_save_custom_fields_user_profile'); add_action('personal_options_update', 'dev_save_custom_fields_user_profile'); function dev_save_custom_fields_user_profile($user_id) { $meta_title = $_POST['meta_title']; update_user_meta($user_id, 'meta_title', $meta_title); }