How to check RAM and CPU in Laravel
This tutorial is on How to check the ram and CPU in Laravel in the Ubuntu operating system(OS). many times clients require to check how much RAM and CPU usage of consumption is in the server. if we do have not to code then we need to check manually every time, so I will give you an example with code that can get data on RAM and CPU usage in your admin panel.
<?php # app/Http/Controllers/HomeController.php namespace App\Http\Controllers; use Illuminate\Http\Request; class HomeController extends Controller { public function total_ram_cpu() { # RAM usage $free = shell_exec('free'); $free = (string) trim($free); $arr_free = explode("\n", $free); $memorys = explode(" ", $arr_free[1]); $memorys = array_filter($memorys); $memorys = array_merge($memorys); $used_memorys = $memorys[2]; $used_memory_in_gb = number_format($used_memorys / 1048576, 2) . ' GB'; $memory_first = $memorys[2] / $memorys[1] * 100; $memory = round($memory_first) . '%'; $fh = fopen('/proc/meminfo', 'r'); $memory_count = 0; while ($line = fgets($fh)) { $piece = array(); if (preg_match('/^MemTotal:\s+(\d+)\skB$/', $line, $piece)) { $memory_count = $piece[1]; break; } } fclose($fh); $total_ram = number_format($memory_count / 1048576, 2) . ' GB'; # cpu usage $cpu_loaded = sys_getloadavg(); $load_width = $cpu_loaded[0]; $load = $cpu_loaded[0] . '% / 100%'; return view('display',compact('memory','total_ram','used_memory_in_gb','load','load_width')); } }
And create display.blade.php
and add below code.
# resources/views/display.blade.php <html> <head> <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css" rel="stylesheet"> <title>How to check ram and cpu in laravel</title> </head> <body> <div class="col-md-3"> <h2 class="no-margin text-semibold">RAM Usage(Current)</h2> <div class="progress progress-micro mb-10"> <div class="progress-bar bg-indigo-400" style="width:{{$memory}}"> <span class="sr-only">{{$memory}}</span> </div> </div> <span class="pull-right">{{$used_memory_in_gb}} / {{$total_ram}} ({{$memory}})</span> </div> <div class="col-md-3"> <h2 class="no-margin text-semibold">CPU Usage(Current)</h2> <div class="progress progress-micro mb-10"> <div class="progress-bar bg-indigo-400" style="width:{{$load_width}}"> <span class="sr-only">{{$load}}</span> </div> </div> <span class="pull-right">{{$load}}</span> </div> </body> </html>
Now route define.
#routes/web.php Route::get('ram_cpu', 'StudentController@total_ram_cpu');
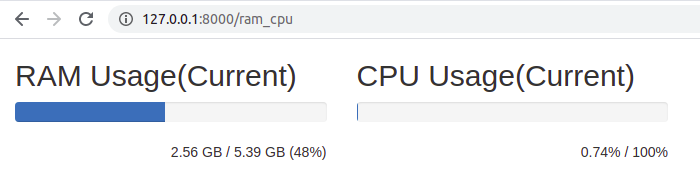
undefined offset: 1 laravel, how to resolve that?