How to Create a Cookie Consent Popup in JavaScript
Today, we will learn How to Create a Cookie Consent Popup in JavaScript. In this tutorial, you will learn how to create a simple Cookie Consent Popup in JavaScript, HTML & CSS. The cookie consent popup box on various websites asks to accept their cookies policy. Cookies store the user information as a text file in the web browser. So when users come back to the website, they will easily get the relevant information.
Also read: How to add GDPR Cookie Consent in Laravel
When users come to your website, they will get a cookie consent popup and accept the cookies policy. Cookie Consent is what users must know about the cookie privacy & policy of your website.
Create a Cookie Consent Popup in JavaScript
<!DOCTYPE html>
<html>
<head>
<meta charset='utf-8'>
<meta http-equiv='X-UA-Compatible' content='IE=edge'>
<title>How to Create a Cookie Consent Popup in JavaScript</title>
<meta name='viewport' content='width=device-width, initial-scale=1'>
<style>
#cookieConcentPopup {
background: white;
width: 25%;
position: fixed;
left: 10px;
bottom: 20px;
box-shadow: 0px 0px 15px #cccccc;
padding: 10px;
border-radius: 10px;
}
#cookieConcentPopup h3 {
margin: 0;
}
#cookieConcentPopup p {
text-align: left;
font-size: 17px;
color: #4e4e4e;
}
#cookieConcentPopup button {
width: 100%;
border: 1px solid #097fb7;
background: #097fb7;
padding: 5px;
border-radius: 8px;
color: white;
}
#acceptCookieConcent {
cursor: pointer;
}
</style>
</head>
<body>
<div id="cookieConcentPopup">
<h3>Cookie Consent</h3>
<p>Lorem ipsum dolor sit amet consectetur adipisicing elit. Velit ducimus, doloremque eligendi animi minus reiciendis perferendis exercitationem soluta ut dolorum nemo corrupti, praesentium asperiores cumque qui earum. Voluptas, ipsam sint? <a href="#">Cookie Policy & Privacy</a></p>
<button id="acceptCookieConcent">Accept</button>
</div>
<script type="text/javascript">
var c_name= "Devnote";
var c_value="Devnote Tutorials";
var c_expire_days= 15;
let acceptCookieConcent = document.getElementById("acceptCookieConcent");
acceptCookieConcent.onclick= function() {
createCookie(c_name, c_value, c_expire_days);
}
// function to set cookie in web browser
let createCookie= function(c_name, c_value, c_expire_days) {
let todayDate = new Date();
todayDate.setTime(todayDate.getTime() +
(c_expire_days*24*60*60*1000));
let expires = "expires=" + todayDate.toGMTString();
document.cookie = c_name + "=" + c_value + ";" + expires + ";path=/";
if(document.cookie) {
document.getElementById("cookieConcentPopup").style.display = "none";
} else {
alert("Unable to set cookie. Please check your cookies setting");
}
}
// get cookie from the web browser
let getCookie= function(c_name) {
let name = c_name + "=";
let decodedCookie = decodeURIComponent(document.cookie);
let ca = decodedCookie.split(';');
for(let i = 0; i < ca.length; i++) {
let c = ca[i];
while (c.charAt(0) == ' ') {
c = c.substring(1);
}
if (c.indexOf(name) == 0) {
return c.substring(name.length, c.length);
}
}
return "";
}
// check cookie is set or not
let checkCookie= function() {
let check=getCookie(c_name);
if(check=="") {
document.getElementById("cookieConcentPopup").style.display = "block";
} else {
document.getElementById("cookieConcentPopup").style.display = "none";
}
}
checkCookie();
</script>
</body>
</html>
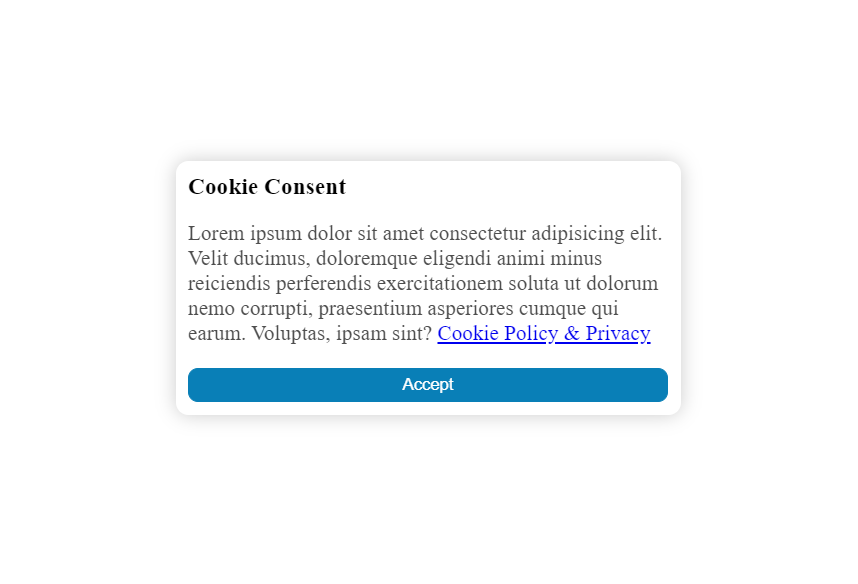