How to create a Livewire Image Upload in Laravel
Today we will create How to create a Livewire Image Upload in Laravel. I will give a very simple example of laravel livewire Image upload tutorial. When the user implements a laravel livewire image upload, he gets some error. So In this tutorial, I will give simple examples of How to create a Livewire Image upload form.
Also, You can use laravel livewire Image upload with laravel 6, laravel 7, laravel 8 and laravel 9 versions.
In this tutorial, I will give you a simple example of creating a contact form with a name and email and I will store that data in the database without refreshing the page. we will use livewire/livewire
the package.
In this tutorial, I will give you a very simple example of creating an image upload form with a name and then I will store data in the database without refreshing the page.
How to create a Livewire Image Upload in Laravel
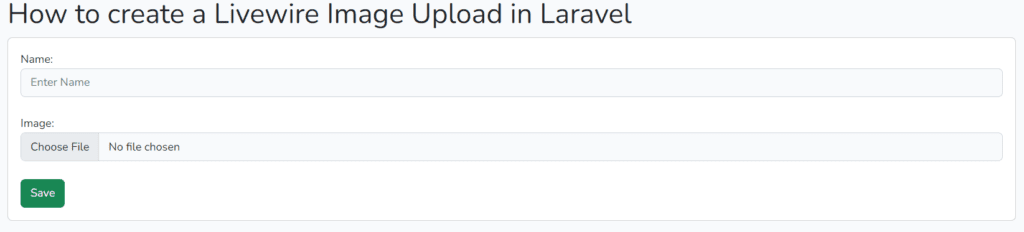
Table of concept
- Install Livewire
- Create Migration and Model
- Create Component
- Create a Route
- Create a View File
1. Install Livewire
Also read: How to create Livewire Pagination in Laravel step 1.
2. Create Migration and Model
In this step, We can create a database migration for the student table and also we will create a model for the student’s table. So let’s start:
php artisan make:model Student -m

Migration File
Also read: How to Upload Profile in Registration Form in Laravel?
<?php
#database\migrations/2023_03_07_124121_create_students_table.php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('students', function (Blueprint $table) {
$table->id();
$table->string('name');//Added
$table->string('image');//Added
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('students');
}
};
And run the below command:
php artisan migrate
Model File
<?php
#app/Models/Student.php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Student extends Model
{
use HasFactory;
/**
* Write code on Method
*
* @return response()
*/
protected $fillable = [
'name', 'image' // Added
];
}
3. Create Component
Now, we will create a livewire component using the below command.
php artisan make:livewire image-upload
The above command creates a contact form component. and they created two files:
Also read: How to create a Livewire Form in Laravel
CLASS: app/Http/Livewire//ImageUpload.php
VIEW: E:\xampp\htdocs\practise\resources\views/livewire/image-upload.blade.php
Now both file we will update both as the below code.
<?php
#app/Http/Livewire/ImageUpload.php
namespace App\Http\Livewire;
use Livewire\Component;
use Livewire\WithFileUploads;
use App\Models\Student;
class ImageUpload extends Component
{
use WithFileUploads;
public $name, $image;
/* Added */
public function submit()
{
$validatedData = $this->validate([
'name' => 'required|unique:students',
'image' => 'required|image|mimes:jpeg,png,jpg|max:2048',
]);
$validatedData['image'] = $this->image->store('images', 'public');
Student::create($validatedData);
session()->flash('message', 'Student successfully created.');
}
public function render()
{
return view('livewire.image-upload');
}
}
resources/views/livewire/image-upload.blade.php
<form wire:submit.prevent="submit" enctype="multipart/form-data">
<div>
@if(session()->has('message'))
<div class="alert alert-success">
{{ session('message') }}
</div>
@endif
</div>
<div class="form-group">
<label for="name">Name:</label>
<input type="text" class="form-control" id="name" placeholder="Enter Name" wire:model="name">
@error('name')
<span class="text-danger">{{ $message }}</span>
@enderror
</div>
<br>
<div class="form-group">
<label for="image">Image:</label>
<input type="file" class="form-control" id="image" wire:model="image">
@error('image')
<span class="text-danger">{{ $message }}</span>
@enderror
</div>
<br>
<button type="submit" class="btn btn-success">Save</button>
</form>
4. Create a Route
Now we will create one route for calling our example.
#routes/web.php
Route::get('student-form', function () {
return view('student.create');
});
5. Create a View File
Last, we will create a blade file for the call form route. in this file, we will use @livewireStyles and @livewireScripts.
resources/views/student/create.blade.php
<!DOCTYPE html>
<html>
<head>
<title>How to create a Livewire Image Upload in Laravel</title>
@vite(['resources/sass/app.scss', 'resources/js/app.js'])
</head>
<body>
<div class="container">
<h1>How to create a Livewire Image Upload in Laravel</h1>
<div class="card">
<div class="card-body">
@livewire('image-upload')
</div>
</div>
</div>
</body>
@livewireScripts
</html>