How to create laravel backup and display it
Backups is most important of your project and database. In this tutorial I will cover how to create backup and it backup how to display, with Spatie’s backup package.
Installation
We’ll installing Spaties laravel backup package :
$ composer require spatie/laravel-backup
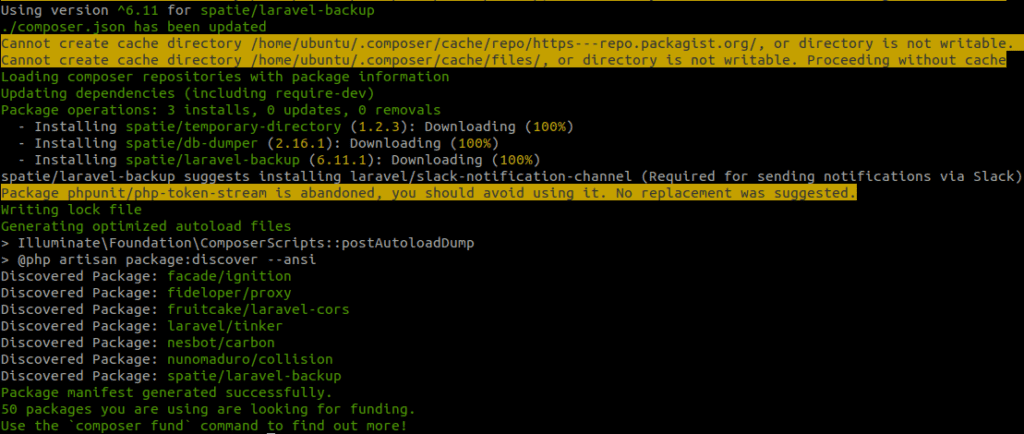
Register the service provider
#config/app.php 'providers' => [ ... Spatie\Backup\BackupServiceProvider::class, ]
The config file publish:
$ php artisan vendor:publish --provider="Spatie\Backup\BackupServiceProvider"

Schedule it in commands kernel :
$schedule->command('backup:clean')->dailyAt('02:50');
$schedule->command('backup:run --only-db')->dailyAt('03:00');
show backup
Here, i will show all backup and it donwload and deleted button display. so bellow code insert your laravel project.
First check you project in storage folder is create or not if not create so run bellow command:
$ php artisan storage:link

Storage folder is wepapp cannot access usually links with your public folder. So your app can access the pictures.
Create Controller
$ php artisan make:controller BackupController
#app/Http/Controllers/BackupController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Artisan;
use Log;
use Session;
use Illuminate\Support\Facades\Storage;
class BackupController extends Controller{
public function index(){
$disk = Storage::disk(config('laravel-backup.backup.destination.disks')[0]);
$files = $disk->files('/Backup_folder_name/');
$backups = [];
foreach ($files as $k => $f) {
if (substr($f, -4) == '.zip' && $disk->exists($f)) {
$backups[] = [
'file_path' => $f,
'file_name' => str_replace(config('laravel-backup.backup.name') . 'Backup_folder_name/', '', $f),
'file_size' => $disk->size($f),
'last_modified' => $disk->lastModified($f),
];
}
}
$backups = array_reverse($backups);
return view("backup.backups")->with(compact('backups'));
}
public static function humanFileSize($size,$unit="") {
if( (!$unit && $size >= 1<<30) || $unit == "GB")
return number_format($size/(1<<30),2)."GB";
if( (!$unit && $size >= 1<<20) || $unit == "MB")
return number_format($size/(1<<20),2)."MB";
if( (!$unit && $size >= 1<<10) || $unit == "KB")
return number_format($size/(1<<10),2)."KB";
return number_format($size)." bytes";
}
public function create()
{
try {
/* only database backup*/
Artisan::call('backup:run --only-db');
/* all backup */
/* Artisan::call('backup:run'); */
$output = Artisan::output();
Log::info("Backpack\BackupManager -- new backup started \r\n" . $output);
session()->flash('success', 'Successfully created backup!');
return redirect()->back();
} catch (Exception $e) {
session()->flash('danger', $e->getMessage());
return redirect()->back();
}
}
public function download($file_name) {
$file = config('laravel-backup.backup.name') .'/Backup_folder_name/'. $file_name;
$disk = Storage::disk(config('laravel-backup.backup.destination.disks')[0]);
if ($disk->exists($file)) {
$fs = Storage::disk(config('laravel-backup.backup.destination.disks')[0])->getDriver();
$stream = $fs->readStream($file);
return \Response::stream(function () use ($stream) {
fpassthru($stream);
}, 200, [
"Content-Type" => $fs->getMimetype($file),
"Content-Length" => $fs->getSize($file),
"Content-disposition" => "attachment; filename=\"" . basename($file) . "\"",
]);
} else {
abort(404, "Backup file doesn't exist.");
}
}
public function delete($file_name){
$disk = Storage::disk(config('laravel-backup.backup.destination.disks')[0]);
if ($disk->exists(config('laravel-backup.backup.name') . '/Backup_folder_name/' . $file_name)) {
$disk->delete(config('laravel-backup.backup.name') . '/Backup_folder_name/' . $file_name);
session()->flash('delete', 'Successfully deleted backup!');
return redirect()->back();
} else {
abort(404, "Backup file doesn't exist.");
}
}
}
Note : Trying to access array offset on value of type bool error is going. so you can change bellow code.
Change this functions index,download,delete.
#old $disk = Storage::disk(config('laravel-backup.backup.destination.disks')[0]);
#new $disk = Storage::disk(config('laravel-backup.backup.destination.disks'));
Create views file
#resources/views/backup/backups.blade.php
<!doctype html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- CSRF Token -->
<link rel="shortcut icon" href="{{ assert('favicon.ico') }}" />
<meta name="csrf-token" content="{{ csrf_token() }}">
<title>{{ config('app.name', 'Laravel') }}</title>
<link type="text/css" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" rel="stylesheet" />
</head>
<body>
<h3>Database Backups</h3>
<div class="row">
<div class="col-xs-12 clearfix">
<form action="{{ url('backup/create') }}" method="GET" class="add-new-backup" enctype="multipart/form-data" id="CreateBackupForm">
{{ csrf_field() }}
<input type="submit" name="submit" class="theme-button btn btn-primary pull-right" style="margin-bottom:2em;" value="Create Database Backup">
</form>
</div>
<div class="col-xs-12">
@if ( Session::has('success') )
<div class="alert alert-success alert-dismissible">
<button type="button" class="close" data-dismiss="alert">×</button>
{{ Session::get('success') }}
</div>
@endif
@if ( Session::has('update') )
<div class="alert alert-success alert-dismissible">
<button type="button" class="close" data-dismiss="alert">×</button>
{{ Session::get('update') }}
</div>
@endif
@if ( Session::has('delete') )
<div class="alert alert-danger alert-dismissible">
<button type="button" class="close" data-dismiss="alert">×</button>
{{ Session::get('delete') }}
</div>
@endif
@if (count($backups))
<table class="table table-striped table-bordered">
<thead>
<tr>
<th>File Name</th>
<th>File Size</th>
<th>Created Date</th>
<th>Created Age</th>
<th>Action</th>
</tr>
</thead>
<tbody>
@foreach($backups as $backup)
<tr>
<td>{{ $backup['file_name'] }}</td>
<td>{{ \App\Http\Controllers\BackupController::humanFilesize($backup['file_size']) }}</td>
<td>
{{ date('F jS, Y, g:ia (T)',$backup['last_modified']) }}
</td>
<td>
{{ \Carbon\Carbon::parse($backup['last_modified'])->diffForHumans() }}
</td>
<td class="text-right">
<a class="btn btn-success"
href="{{ url('backup/download/'.$backup['file_name']) }}"><i
class="fa fa-cloud-download"></i> Download</a>
<a class="btn btn-danger" onclick="return confirm('Do you really want to delete this file')" data-button-type="delete"
href="{{ url('backup/delete/'.$backup['file_name']) }}"><i class="fa fa-trash-o"></i>
Delete</a>
</td>
</tr>
@endforeach
</tbody>
</table>
@else
<div class="well">
<h4>No backups</h4>
</div>
@endif
</div>
</div>
<script src="https://code.jquery.com/jquery-3.5.1.min.js" type="text/javascript"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js" type="text/javascript"></script>
<script type="text/javascript">
$("#CreateBackupForm").on('submit',function(e){
$('.theme-button').attr('disabled','disabled');
});
</script>
</body>
</html>
Route define
#routes/web.php Route::get('/backup', 'BackupController@index'); Route::get('/backup/create', 'BackupController@create'); Route::get('/backup/download/{file_name}', 'BackupController@download'); Route::get('/backup/delete/{file_name}', 'BackupController@delete');
do I need to change this line? what is this line means
$disk = Storage::disk(config(‘laravel-backup.backup.destination.disks’)[0]);
$files = $disk->files(‘/Backup_folder_name/’);
Let’s break down the two lines and explain their purpose:
1. $disk = Storage::disk(config(‘laravel-backup.backup.destination.disks’)[0]);
Purpose:
This line is creating a $disk instance that corresponds to a specific storage disk in Laravel.
Breakdown:
config(‘laravel-backup.backup.destination.disks’):):
Retrieves the array of disk names configured in the laravel-backup package’s configuration file (likely found in config/laravel-backup.php under the backup.destination.disks key).
[0]:
Gets the first disk name from the array.
Storage::disk(
Creates a storage instance for the specified disk, allowing you to interact with files stored on that disk.
2. $files = $disk->files(‘/Backup_folder_name/’);
Purpose:
Retrieves the list of files from the /Backup_folder_name/ directory on the specified disk.
Breakdown:
$disk->files(‘‘): on the disk represented by $disk.
Fetches all files from the specified directory