How to create Livewire Datatables Using Laravel
Today I will give you an example of How to create Livewire Datatables Using Laravel. In this tutorial, we will create livewire datatables using laravel with MedicOneSystems/livewire-datatables package. If you want to see an example of how to create livewire datatable in Laravel then you are in the right place.
Livewire Datatable is a full-stack framework for Laravel that makes building dynamic interfaces simple, without leaving the comfort of Laravel. if you are using livewire with laravel then you don’t worry about writing jquery ajax code and livewire will help to write a very simple jquery ajax code.
Here, I will give an example of creating data tables with a users table and I will display data using datatables. I will use only livewire/livewire package.
Also read: How to create Livewire Pagination in Laravel
How to create Livewire Datatables Using Laravel Preview
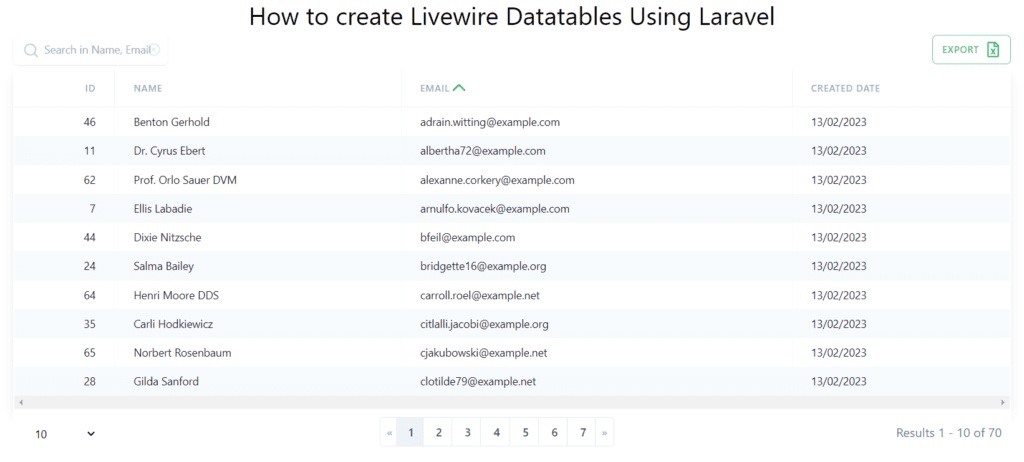
Table of concept
- Create Dummy Records
- Install Livewire & livewire-datatables Package
- Define Route
- Create View File
- Run Laravel Project
1. Create Dummy Records
You need to run the below command to create dummy records in your user’s table.
php artisan tinker
App\Models\User::factory()->count(70)->create();
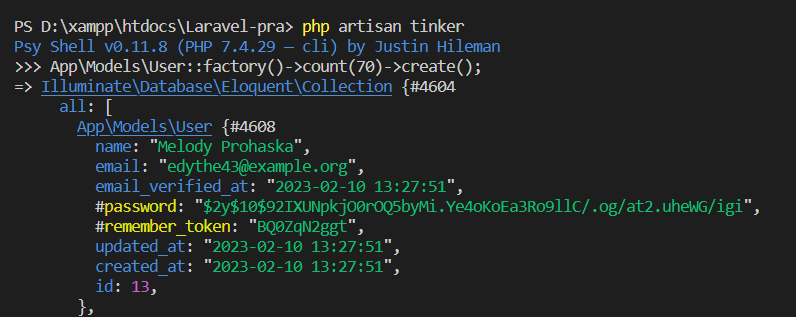
2. Install Livewire & livewire-datatables Package
In this step, we will install livewire to our laravel application using the below command:
composer require livewire/livewire
composer require mediconesystems/livewire-datatables
Also, we will create a livewire component using the below command:
php artisan make:livewire user-datatables
The above command created two files:
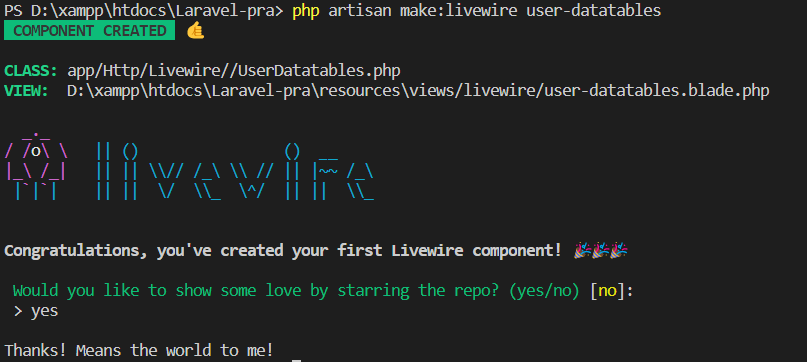
Now we will update the Class file.
<?php
#app/Http/Livewire/UserDatatables.php
namespace App\Http\Livewire;
use Livewire\Component;
use App\Models\User; //Added
use Illuminate\Support\Str; //Added
use Mediconesystems\LivewireDatatables\Column; //Added
use Mediconesystems\LivewireDatatables\NumberColumn; //Added
use Mediconesystems\LivewireDatatables\DateColumn; //Added
use Mediconesystems\LivewireDatatables\Http\Livewire\LivewireDatatable; //Added
class UserDatatables extends LivewireDatatable // Added
{
public $model = User::class; // Added
public function columns() // Added
{
return [
NumberColumn::name('id'),
Column::name('name'),
Column::name('email'),
DateColumn::name('created_at')->label('Created Date')
];
}
}
3. Define Route
Also read: How to create a Livewire Form in Laravel
Now we will create one route for calling our example, so add the bellow code in the web.php file:
<?php
#web.php
Route::get('user-data', function () {
return view('users.index');
});
4. Create a View File
resources/views/users/index.blade.php
<!DOCTYPE html>
<html>
<head>
<title>How to create Livewire Datatables Using Laravel</title>
@livewireStyles
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/tailwindcss/1.9.2/tailwind.min.css">
<style>
h1 {
font-size: 32px;
}
.container {
text-align: center;
margin: 0 auto;
}
</style>
</head>
<body>
<div class=" container">
<h1>How to create Livewire Datatables Using Laravel</h1>
<livewire:user-datatables searchable="name, email" exportable />
</div>
</body>
@livewireScripts
</html>
5. Run Laravel Project
Finally, the required steps have been done, So you can run the below command:
php artisan serve
And then Go to your web browser and type the below URL:
http://127.0.0.1:8000/user-data