How to inline row editing using Laravel
In this tutorial, we will learn How to inline row editing using Laravel. Here tutorial will give you a simple example of Laravel ajax inline edit row and you will learn Laravel x-editable example. I will show you how to simple example of how to create table inline editing in Laravel application and you can use this example with Laravel 8. we will display a list of students and you can edit his name and emails using x-editable js.
- Install Laravel
- Create Controller
- Define Route
- Create Blade File
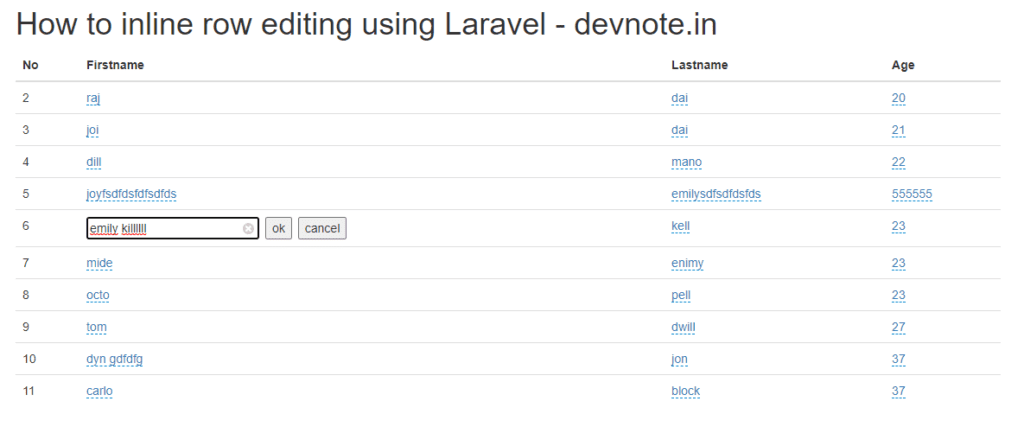
Install Laravel
first of all we need to get install Laravel 8 application using bellow command:
composer create-project --prefer-dist laravel/laravel blog
Also read: Laravel 8 CRUD Operation Tutorial for Beginners
Create Controller
We need to create StudentController
and add following code:
<?php
#app/Http/Controllers/StudentController.php
namespace App\Http\Controllers;
use App\Models\Student;
use Illuminate\Http\Request;
class StudentController extends Controller
{
public function index()
{
$students = Student::get();
return view('student',compact('students'));
}
public function update(Request $request)
{
if ($request->ajax()) {
Student::find($request->pk)->update([
$request->name => $request->value
]);
return response()->json(['success' => true]);
}
}
}
Define Route
We need to define some routes for add and delete function.
<?php
#routes/web.php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\StudentController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('student', [StudentController::class, 'index'])->name('student.index');
Route::post('student', [StudentController::class, 'update'])->name('student.update');
Create Blade File
We need to create blade files for students data display. so let’s create files:
resources/views/students.blade.php
students.blade.php
<!DOCTYPE html>
<html>
<head>
<title>How to inline row editing using Laravel - devnote.in</title>
<meta name="csrf-token" content="{{ csrf_token() }}">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css" />
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<link href="https://cdnjs.cloudflare.com/ajax/libs/x-editable/1.5.0/jquery-editable/css/jquery-editable.css" rel="stylesheet"/>
<script>$.fn.poshytip={defaults:null}</script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/x-editable/1.5.0/jquery-editable/js/jquery-editable-poshytip.min.js"></script>
</head>
<body>
<div class="container">
<h1>How to inline row editing using Laravel - devnote.in</h1>
<table class="table table-border data-table">
<thead>
<tr>
<th>No</th>
<th>Firstname</th>
<th>Lastname</th>
<th>Age</th>
</tr>
</thead>
<tbody>
@foreach ($students as $student)
<tr>
<td>{{ $student->id }}</td>
<td>
<a href="" class="update_record" data-name="firstname" data-type="text" data-pk="{{ $student->id }}" data-title="Enter Firstname">{{ $student->firstname }}</a>
</td>
<td>
<a href="" class="update_record" data-name="lastname" data-type="text" data-pk="{{ $student->id }}" data-title="Enter Lastname">{{ $student->lastname }}</a>
</td>
<td>
<a href="" class="update_record" data-name="age" data-type="text" data-pk="{{ $student->id }}" data-title="Enter Age">{{ $student->age }}</a>
</td>
</tr>
@endforeach
</tbody>
</table>
</div>
</body>
<script type="text/javascript">
$.fn.editable.defaults.mode = 'inline';
$.ajaxSetup({
headers: {
'X-CSRF-TOKEN': '{{csrf_token()}}'
}
});
$('.update_record').editable({
url: "{{ route('student.update') }}",
type: 'text',
name: 'firstname',
pk: 1,
title: 'Enter Field'
});
</script>
</html>
Now, we are ready to run our example:
php artisan serve
Now open bellow URL on your browser:
localhost:8000/student