How to integrate Google reCAPTCHA v3 in PHP
This tutorial is on How to integrate Google reCAPTCHA v3 in PHP. you have used third-party anti-spam solutions for your website. here show you how to add Google reCAPTCHA v3 to a form on a PHP website. we’ll discuss reCAPTCHA v3, which is invisible and doesn’t require user interaction at all. Google reCAPTCHA v3 works on the basis of spam score and the reCAPTCHA v3 API returns the spam score of each request given by the user activity.
Get the site key and secret key
Go to Admin console. Click the right side plus sign(+).
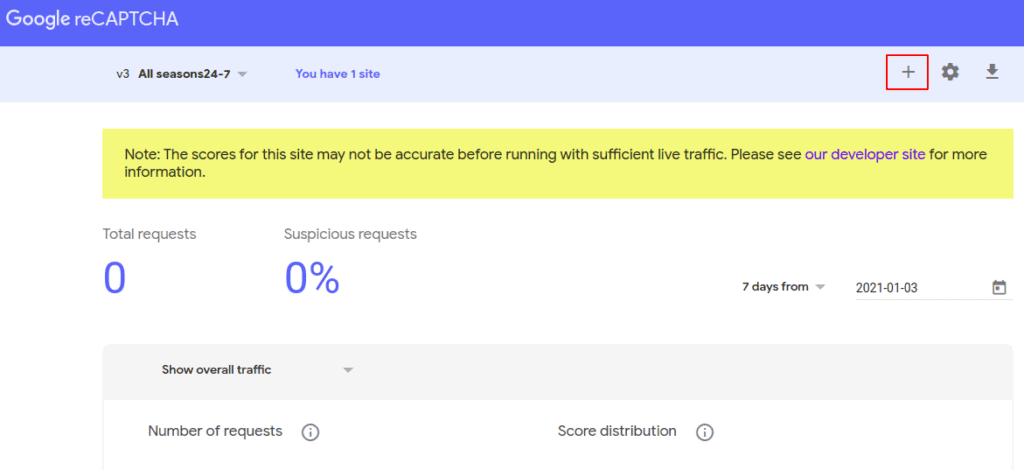
Label
Using a label it can easy for you to identify the site in the future.
reCAPTCHA type
The site key only works with a single reCAPTCHA site type. see Site Types for more details.
Add domains
A registration for yourdomain.com also registers subdomain.yourdomain.com.
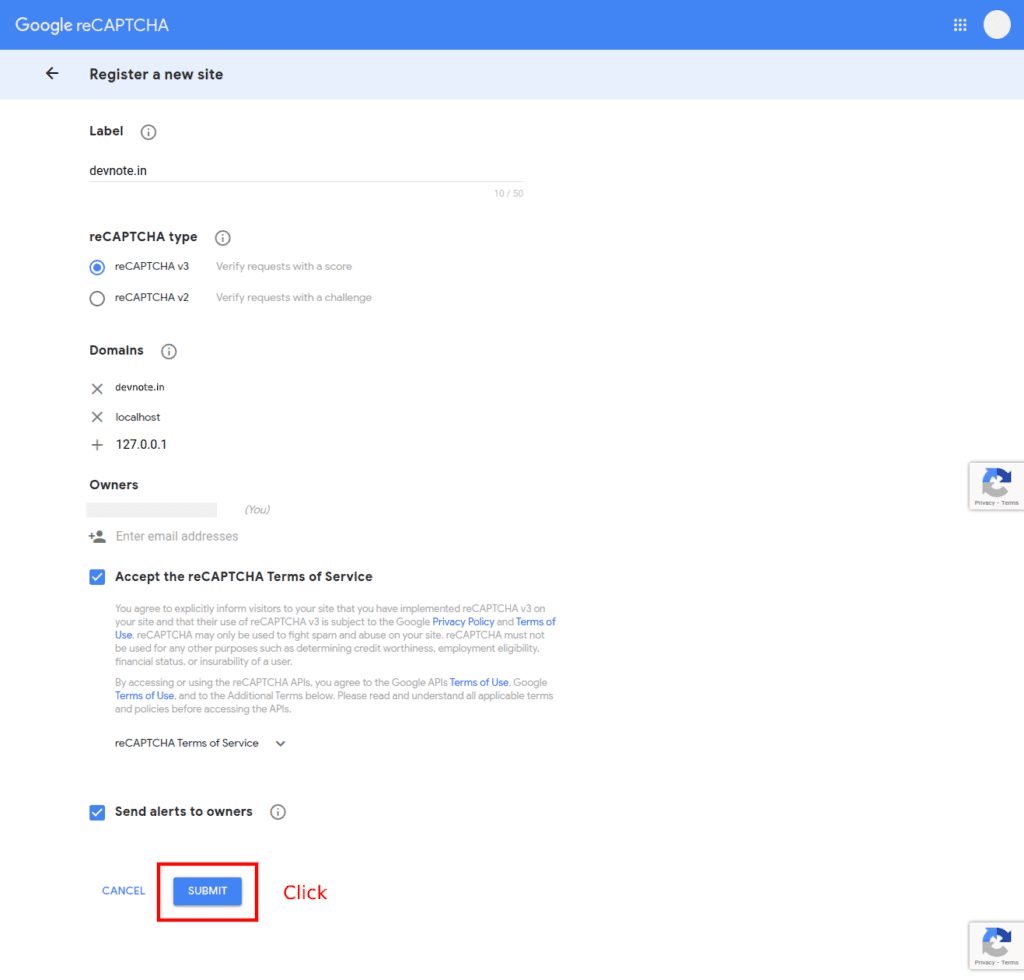
Finally submit the form and You will get the site key and secret key that you can use in the code.
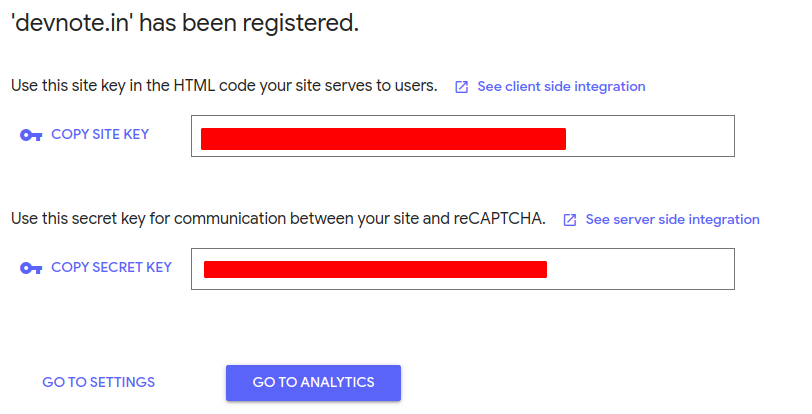
Integration Google reCAPTCHA v3 in PHP and Ajax
Integrate Google reCAPTCHA v3 using two sides use first client and the second server side.
Index.php
<!DOCTYPE html>
<html>
<head>
<title>How to integrate Google reCAPTCHA v3 in PHP</title>
<style>
body{
text-align: center;
}
</style>
</head>
<body>
<h1>How to integrate Google reCAPTCHA v3 in PHP</h1>
<form id="google_recaptcha">
Name : <input type="text" id="name" name="name"/><br><br>
Mobile Number : <input type="text" id="number" name="number"/><br><br>
<input type="submit" name="submit" value="Submit" class="submitbtn">
</form>
<script src="https://code.jquery.com/jquery-3.4.1.min.js"></script>
<!-- JavaScript API with your sitekey. -->
<script src="https://www.google.com/recaptcha/api.js?render=put-your-site-key"></script>
<script type="text/javascript">
$(document).on('submit', '#google_recaptcha', function(e){
e.preventDefault();
grecaptcha.ready(function() {
// grecaptcha.execute on each action
grecaptcha.execute('put-your-site-key', {action: 'application_form'}).then(function(token) {
formDatas = {
name : $('#name').val(),
number : $('#number').val(),
token: token,
action : 'application_form'
};
$.post('captchacheck.php', formDatas, function(response){
alert(response);
});
});
});
});
</script>
</body>
</html>
captchacheck.php
<?php
if (!empty($_POST)) { # Direct not open this file
$name = $_POST['name'];
$number = $_POST['number'];
$token = $_POST['token']; // Use for google reCAPTCHA execute
$action = $_POST['action']; // Use security purpose and
if ($name != "" && $number != "") {
$curl_data = array(
'secret' => 'put_your_secret_key',
'response' => $token
);
# Initialize a cURL session
$curlInit = curl_init();
curl_setopt($curlInit, CURLOPT_URL, "https://www.google.com/recaptcha/api/siteverify");
curl_setopt($curlInit, CURLOPT_POST, 1);
curl_setopt($curlInit, CURLOPT_POSTFIELDS, http_build_query($curl_data));
curl_setopt($curlInit, CURLOPT_RETURNTRANSFER, true);
$curl_response = curl_exec($curlInit);
$captcha_code_response = json_decode($curl_response, true);
if($captcha_code_response['success'] == '1'
&& $captcha_code_response['action'] == $action
&& $captcha_code_response['score'] >= 0.5
&& $captcha_code_response['hostname'] == $_SERVER['SERVER_NAME']) {
echo 'Your request successfully have been send!';
} else {
echo 'You are not a human!';
}
} else {
echo 'Please fillup field!';
}
} else {
header("location:index.php");
}
Output
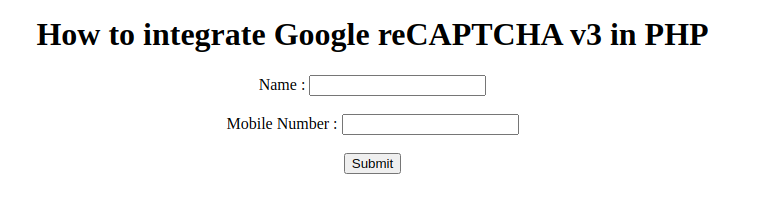
This tutorial is really good with an explanation. You can also check another article to add Google reCAPTCHA V3 in PHP Ajax contact form with a live demo and can download complete code.
https://flamontech.com/google-recaptcha-v3-php-ajax-example-github-link/
Thank you.