How to take input from the console in python
This tutorial is on How to take input from the console in python. Console (also called Shell) is basically a command line interpreter(CLI) that takes input from the user i.e one command at a time and interprets it. A Python Console looks like this.

The python console is the three greater than symbols. >>>
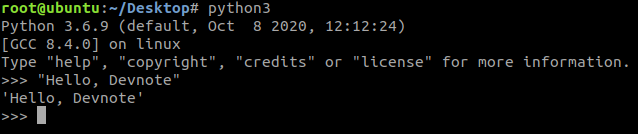
Input from Console
To take input from the user we make use of a python built-in function input(). We can also typecast this input to integer, float, or string by specifying the input() function.
integer
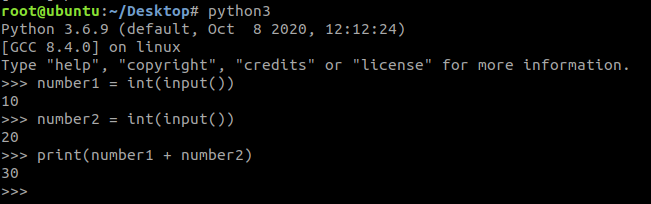
number1 = int(input()) # 10 number2 = int(input()) # 20 print(number1 + number2) Output: 30
float
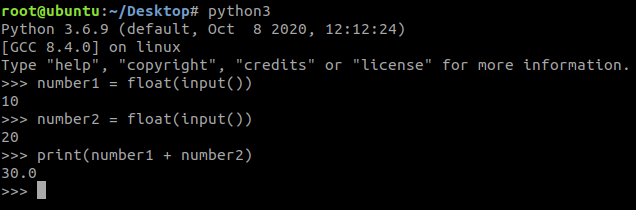
number1 = float(input()) # 10 number2 = float(input()) # 20 print(number1 + number2) Output : 30.0
string
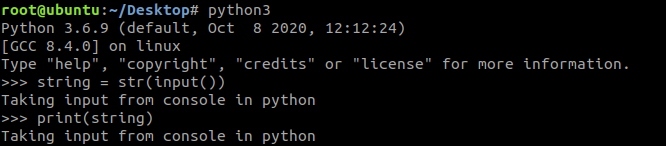
string = str(input())
# Taking input from console in python
print(string)
Output:
Taking input from console in python