How to upload and delete image file using jquery and ajax
This post is for How to upload and delete image files using jquery and ajax. You can easily upload the selected file to your server and show a preview of it. In this tutorial, we will like to add a remove button to delete the file without page load So you again need to use AJAX to remove the file from the server. we will use PHP to handle AJAX requests on the server-side.
Contents
- HTML Code
- PHP Code
- Jquery Script
- Output
- Full code
- Conclusion
HTML Code
First create a <form>
with file and button element and Show the preview with delete button add <div class='upload_image'>
using jQuery.
#index.php
<!DOCTYPE html>
<html>
<head>
<title>How to upload and delete image file using jquery and ajax - devnote.in</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<form method="post" id="uploadForm" enctype="multipart/form-data">
<input type="file" id="user_profile" name="user_profile" />
<input type="button" class="button" value="Upload File" id="btn_upload">
</form>
<div class="upload_image"></div>
</body>
</html>
PHP Code
Create a new ajax.php
file and create user_profile_upload
folder.
<?php #ajax.php if(!empty($_POST)){ $request_status = $_POST['request_status']; if($request_status == 1) { $filename = $_FILES['user_profile']['name']; $file_name = time()."_".$filename; $file_location = "user_profile_upload/".$file_name; $upload_status = 1; $image_type = pathinfo($file_location,PATHINFO_EXTENSION); // Check format if($image_type != "jpg" && $image_type != "png" && $image_type != "gif" ) { $upload_status = 0; } if($upload_status == 0){ echo 0; } else { /* File Upload */ if(move_uploaded_file($_FILES['user_profile']['tmp_name'],$file_location)) { echo $file_location; } else { echo 0; } } exit; } // Remove upload file if($request_status == 2) { $file_path = $_POST['path']; $return_val = 0; if( file_exists($file_path) ){ // Remove file @unlink($file_path); $return_val = 1; } else { $return_val = 0; } echo $return_val; exit; } } else { header("location:index.php"); } ?>
Jquery Script
Click on upload file button click create a FormData
Class object and append the file selected from. and append request_status
with 1 value.
$(document).ready(function(){
$("#btn_upload").click(function(){
var fd = new FormData();
var user_profile = $('#user_profile')[0].files[0];
fd.append('user_profile',user_profile);
fd.append('request_status',1);
// AJAX request
$.ajax({
url: 'ajax.php',
type: 'post',
data: fd,
contentType: false,
processData: false,
success: function(response){
if(response != 0){
var count = $('.upload_image .img_content').length;
count = Number(count) + 1;
// Show image and delete button
$('.upload_image').append("<div class='img_content' id='upload_"+count+"' ><img src='"+response+"' width='100' height='100' style='display: block;margin: 10px 0px 5px 5px;'><span style='cursor: pointer; background: green; padding: 10px 20px;border-radius: 5px;color: #FFF;display: inline-block;margin-top: 10px;margin-left: 10px;' class='delete_img' id='delete_"+count+"'>Delete</span></div>");
} else {
alert('Something went wrong. file not uploaded!');
}
}
});
});
// Remove upload file
$('.upload_image').on('click','.img_content .delete_img',function(){
var id = this.id;
var split_id = id.split('_');
var num = split_id[1];
var img_src = $('#upload_'+num+' img').attr("src");
var delete_file = confirm("Do you really want to Delete this file?");
if (delete_file == true) {
$.ajax({
url: 'ajax.php',
type: 'post',
data: {path: img_src,request_status: 2},
success: function(response){
// Remove image
if(response == 1) {
$('#img_content'+num).remove();
$('#upload_'+num).remove();
$('#user_profile').val('');
}
}
});
}
});
});
Output
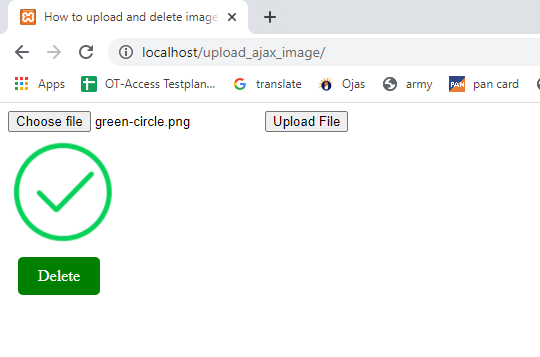
Full code
Conclusion
Use FormData
object variable to send file value and append request_status
using AJAX file for store to the server and with unlink() method to delete the file.