How to use soft delete in Laravel
In this tutorial, we will learn How to use soft delete in Laravel. today you will learn how to use the Laravel soft delete concept. Soft delete is used for deleting records visually which means you can’t see in the front-end, but records are not actually removed from the database. so let’s start soft delete example.
Table of contents
- How to work soft delete
- How to use soft delete
- Soft delete example
How to work soft delete
Soft delete records are not actually removed from the database. soft delete has been assigned to the deleted_at
column and also insert timestamp in this field. And when you get records from the database using soft deletes, then Laravel retrieves those records whose timestamps are nulled in deleted_at
the column.
How to use soft delete
Here, Two simple steps to enable soft delete.
- Add
deletead_at
column in migration file using$table->softDeletes();
- Enable soft deletes in the model file. add the
Illuminate\Database\Eloquent\SoftDeletes
.
And, when you call the delete
method on the model and the deleted_at
column in set the current date and time.
Add softDeletes column in migration
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreatePostsTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('description');
$table->softDeletes(); //Added
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('posts');
}
}
And, Now run the below command to migrate the table.
php artisan migrate
Now, You can get to the database in the posts table and you will show deleted_at
the column.
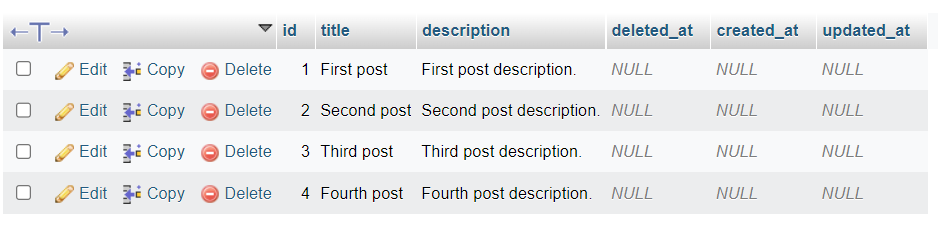
Add softDeletes in the Model file
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\SoftDeletes; // Added
class Posts extends Model
{
use HasFactory, SoftDeletes; //Added
protected $fillable = [
'title', 'description'
];
/**
* The attributes that should be mutated to dates.
*/
protected $dates = ['deleted_at']; // Added
}
soft delete example
When you call the delete method on the model, the deleted_at
column in sets the current timestamp.
soft delete use
$id = 2;
$post = Posts::find($id);
$post->delete();
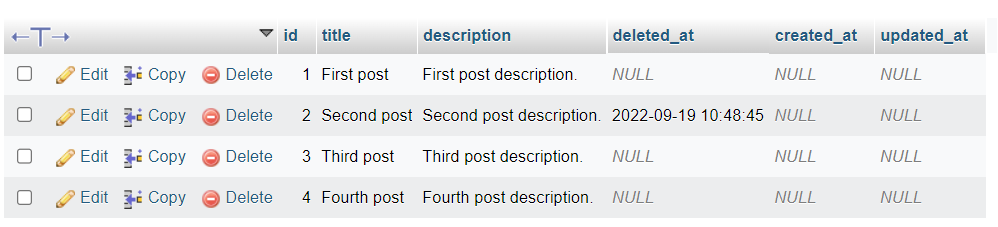