Laravel 8 Authentication example
In this, tutorial we will discuss How to create a Laravel 8 authentication example. In most cases, we need to create an authentication system for keeping our application private. and easily create Laravel 8 authentication. Laravel provides a command to create authentication and built-in solution and various facility to customize it according to your requirements. And if you are new to Laravel 8 then I’ll show you the step by step of making an authentication application in Laravel 8.
Now, Let’s follow the step by step making an authentication application using Laravel 8.
Also read: How to get current user details using Laravel 8
Laravel 8 authentication
- Create a Laravel 8 application
- Install Laravel UI package
- Generate auth scaffold
- Install NPM
- Example
- Disable registration route
- Route restriction
- Change the necessary configuration
Create a Laravel 8 application
Also read: How to Upload Profile in Registration Form in Laravel?
First, we have to create a fresh Laravel 8 application. If you already installed Laravel 8 application then skip this step.
composer create-project --prefer-dist laravel/laravel blog 8.0
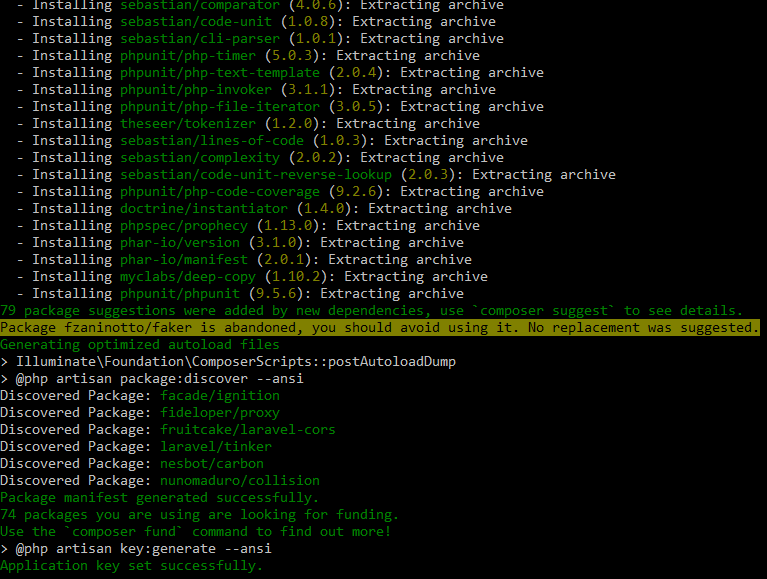
The above command blog is our project name and 8.0 means we install Laravel 8.0 version.
Install Laravel UI package
Install the Laravel UI official package for creating auth scaffolding in the Laravel 8 application.
composer require laravel/ui:^3.0
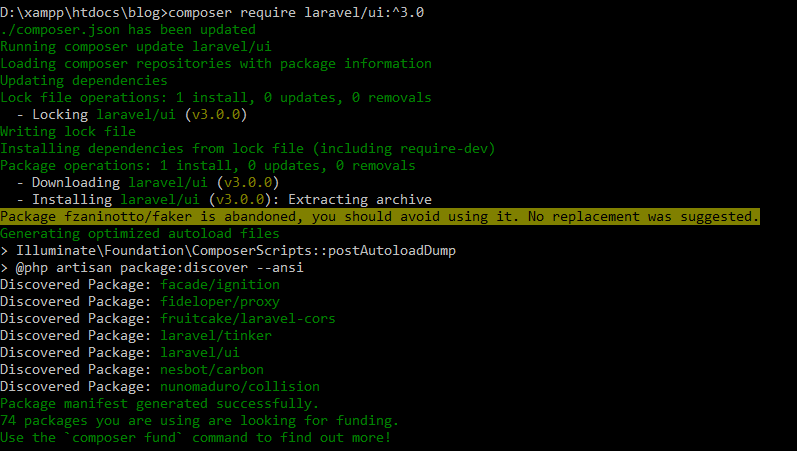
Generate auth scaffold
After installation Laravel UI package. Now, We are able to scaffold our auth with Bootstrap, Vue, React, etc. And if we need to scaffold with Vue then run the command bellow command:
php artisan ui vue --auth
Output
Vue scaffolding installed successfully.
Please run "npm install && npm run dev" to compile your fresh scaffolding.
Authentication scaffolding generated successfully.
Install NPM
Now, we have to install all our NPM dependencies. And compile the assets the command npm run dev
npm install && npm run dev
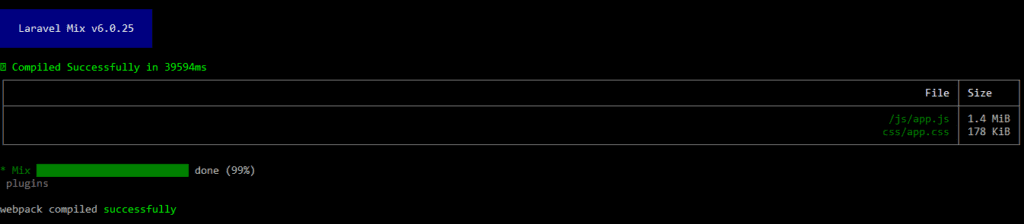
Example
Now ready Laravel 8 auth application. Now check whether authentication is installed or not.
Check login
http://127.0.0.1:8000/login
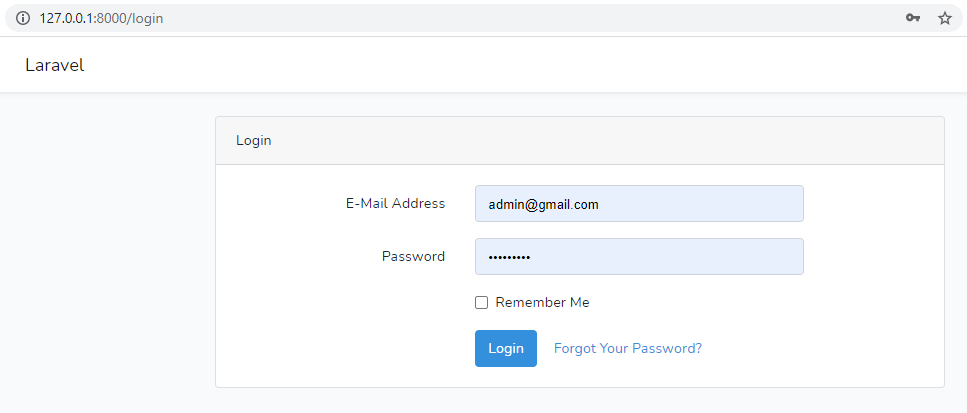
Check registration
http://127.0.0.1:8000/register
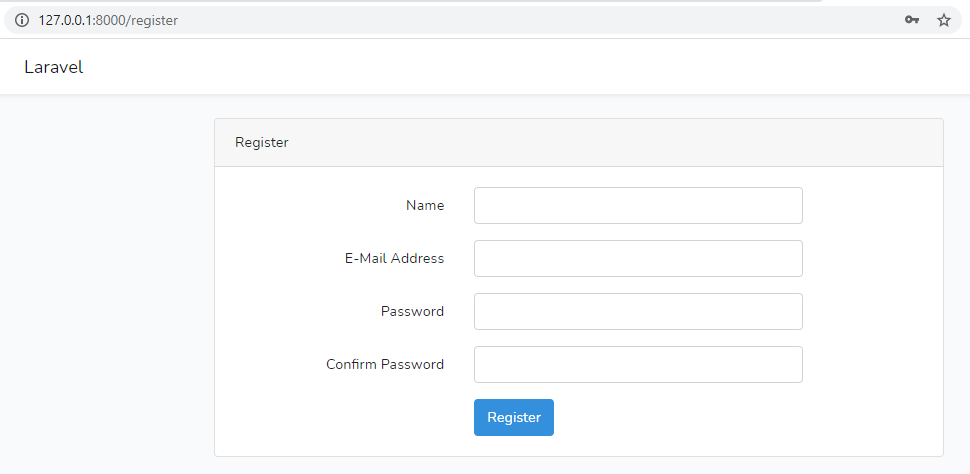
Disable register route
In most cases, we clients want to disable the new user registration system. Then you go to the /routes/web.php route file and add the below code.
Auth::routes(['register' => false]);
Route restriction
Laravel 8 was successfully installed with auth system. Now protect our routes for unauthenticated users by using a two-way routes file and a second controller file.
#routes/web.php
Route::get('/dashboard', 'App\Http\Controllers\DashboardController@index')->middleware('auth');
And we can protect by our controller in the constructor function __construct
<?php
#App\Http\Controllers\DashboardController.php
class UserController extends Controller
{
public function __construct()
{
$this->middleware('auth');
}
public function index(){
// code here
}
}
Now how to check the user is authenticated or not authenticated in the view or anywhere in the controller. so we can use auth()->check()
if(auth()->check()) {
// code here
}
And get current user data.
Also read: How to get current user details using Laravel 8
$user = auth()->user();
Change the necessary configuration
Laravel auth default auth successfully log in it will redirect/home path. but in most cases we can redirect /dashboard or another page so we can put public const HOME=’/dashboard’ form the RouteServiceProvider.php
file.
#App\Providers\RouteServiceProvider.php
public const HOME = '/dashboard';
Now username customization
Also read: How to login with username or email in Laravel authentication
Laravel authentication by default checks the user’s email. and we want to check usernames instead of checking email.
Note: Make sure when you change email to a username then the function name i username
.
#app/Http/Controllers/Auth/LoginController.php
public function username()
{
return 'username';
// code here
}