Laravel 8 CRUD Operation Tutorial for Beginners
This tutorial, Laravel 8 CRUD Operation Tutorial for Beginners
. we will implement a Laravel 8 crud application for beginners. I will simple example of how to create crud in Laravel 8 and you will learn crud operation in Laravel 8.
This tutorial will help you insert, update, delete, and view(read) application in Laravel 8. simple few steps and you will get basic crud operation using controller, model, route, bootstrap 4, and blade.
Step 1: Install Laravel 8
First of all Install Laravel 8 version application using command prompt or terminal.
composer create-project --prefer-dist laravel/laravel laravel_8
Step 2: Database Configuration
#.env ... DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=database_name DB_USERNAME=database_username DB_PASSWORD=database_password ...
Step 3: Create Migration
We are create crud application for student. so create migration for students table using Laravel 8.
php artisan make:migration create_students_table --create=students
#database/migrations/2020_09_28_054835_create_students_table.php
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateStudentsTable extends Migration {
public function up()
{
Schema::create('students', function (Blueprint $table) {
$table->id();
$table->string('firstname');
$table->string('lastname');
$table->integer('age');
$table->timestamps();
});
}
public function down()
{
Schema::dropIfExists('students');
}
}
Now run this migration by following command:
php artisan migrate
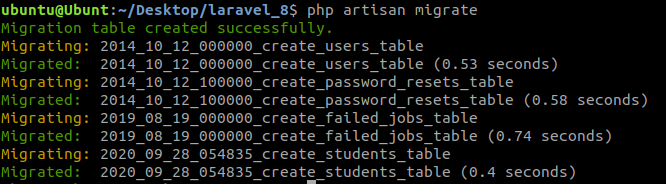
Step 4: Create Controller and Model
Now create new controller as StudentController. Bellow command to create a new controller. This controller is resource controller and it will create seven methods by default provide like (1)index() ,(2)create(), (3)store(), (4)show(), (5)edit(), (6)update(), (7)destroy().
php artisan make:controller StudentController --resource --model=Student
Controller
#app/Http/Controllers/StudentController.php
<?php
namespace App\Http\Controllers;
use App\Models\Student;
use Illuminate\Http\Request;
class StudentController extends Controller
{
public function index()
{
$students = Student::latest()->paginate(10);
return view('students.index',compact('students'))->with('i', (request()->input('page', 1) - 1) * 5);
}
public function create()
{
return view('students.create');
}
public function store(Request $request)
{
$request->validate([
'firstname' => 'required',
'lastname' => 'required',
'age' => 'required',
]);
Student::create($request->all());
return redirect()->route('students.index')->with('success','Student created successfully.');
}
public function show(Student $student)
{
return view('students.show',compact('student'));
}
public function edit(Student $student)
{
return view('students.edit',compact('student'));
}
public function update(Request $request, Student $student)
{
$request->validate([
'firstname' => 'required',
'lastname' => 'required',
'age' => 'required',
]);
$student->update($request->all());
return redirect()->route('students.index')->with('success','Student updated successfully');
}
public function destroy(Student $student)
{
$student->delete();
return redirect()->route('students.index')->with('success','Student deleted successfully');
}
}
Models
#app/Models/Student.php
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Student extends Model
{
use HasFactory;
protected $fillable = ['firstname', 'lastname','age'];
}
Step 5: Create Blade Files
You have to create following bellow blade file:
- layout.blade.php
- index.blade.php
- create.blade.php
- edit.blade.php
- show.blade.php
1) resources/views/students/layout.blade.php
<!DOCTYPE html>
<html>
<head>
<title>Laravel 8 CRUD Operation Tutorial for Beginners</title>
<link href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" rel="stylesheet"/>
</head>
<body>
<div class="container">
@yield('content')
</div>
</body>
</html>
2) resources/views/students/index.blade.php
@extends('students.layout')
@section('content')
<div class="row">
<div class="col-lg-12 margin-tb">
<div class="pull-left text-center">
<h3>Laravel 8 CRUD Operation Tutorial for Beginners</h3>
</div>
<div class="pull-right">
<a class="btn btn-success" href="{{ route('students.create') }}"> Create Student</a>
</div><br>
</div>
</div>
@if ($message = Session::get('success'))
<div class="alert alert-success">
<span>{{ $message }}</span>
</div>
@endif
<table class="table table-bordered">
<tr>
<th>No</th>
<th>Firstname</th>
<th>Lastname</th>
<th>Age</th>
<th>Action</th>
</tr>
@foreach ($students as $student)
<tr>
<td>{{ ++$i }}</td>
<td>{{ $student->firstname }}</td>
<td>{{ $student->lastname }}</td>
<td>{{ $student->age }}</td>
<td><form action="{{ route('students.destroy',$student->id) }}" method="POST">
<a class="btn btn-info" href="{{ route('students.show',$student->id) }}">Show</a>
<a class="btn btn-primary" href="{{ route('students.edit',$student->id) }}">Edit</a>
@csrf
@method('DELETE')
<button type="submit" onclick="return confirm('Do you really want to delete student!')" class="btn btn-danger">Delete</button>
</form></td>
</tr>
@endforeach
</table>
{!! $students->links() !!}
@endsection
3) resources/views/students/create.blade.php
@extends('students.layout')
@section('content')
<div class="row">
<div class="col-lg-12 margin-tb">
<div class="pull-left">
<h2>Create Student</h2>
</div>
</div>
</div>
@if ($errors->any())
<div class="alert alert-danger">
<strong>Whoops!</strong> something we are problems with your input.<br><br>
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
<form action="{{ route('students.store') }}" method="POST">
@csrf
<div class="row">
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Firstname:</strong>
<input type="text" name="firstname" class="form-control" placeholder="First name">
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Lastname:</strong>
<input type="text" name="lastname" class="form-control" placeholder="Last name">
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Age:</strong>
<input type="number" min="1" name="age" class="form-control" placeholder="Age">
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12 text-center">
<a class="btn btn-primary" href="{{ route('students.index') }}"> Back</a>
<button type="submit" class="btn btn-success">Submit</button>
</div>
</div>
</form>
@endsection
4) resources/views/students/edit.blade.php
@extends('students.layout')
@section('content')
<div class="row">
<div class="col-lg-12 margin-tb">
<div class="pull-left">
<h2>Edit Student</h2>
</div>
</div>
</div>
@if ($errors->any())
<div class="alert alert-danger">
<strong>Whoops!</strong> something we are problems with your input.<br><br>
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
<form action="{{ route('students.update',$student->id) }}" method="POST">
@csrf
@method('PUT')
<div class="row">
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Firstname:</strong>
<input type="text" name="firstname" value="{{ $student->firstname }}" class="form-control" placeholder="First name">
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Lastname:</strong>
<input type="text" name="lastname" value="{{ $student->lastname }}" class="form-control" placeholder="Last name">
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Age:</strong>
<input type="number" name="age" min="1" value="{{ $student->age }}" class="form-control" placeholder="Age">
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12 text-center">
<a class="btn btn-primary" href="{{ route('students.index') }}"> Back</a>
<button type="submit" class="btn btn-success">Update</button>
</div>
</div>
</form>
@endsection
5) resources/views/students/show.blade.php
@extends('students.layout')
@section('content')
<br><br>
<div class="row text-center">
<div class="col-lg-12 margin-tb">
<div class="pull-left">
<h3> Student Detail </h3>
</div>
</div>
</div><br>
<div class="row text-center">
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Firstname:</strong>
{{ $student->firstname }}
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Lastname:</strong>
{{ $student->lastname }}
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Age:</strong>
{{ $student->age }}
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<a class="btn btn-primary" href="{{ route('students.index') }}"> Back</a>
</div>
</div>
@endsection
Step 6: Add Route
#routes/web.php use App\Http\Controllers\StudentController; Route::resource('students', StudentController::class);
Now ready to run crud application example with laravel 8.
php artisan serve
Open bellow URL on your browser:
http://localhost:8000/students
You will see layout like:
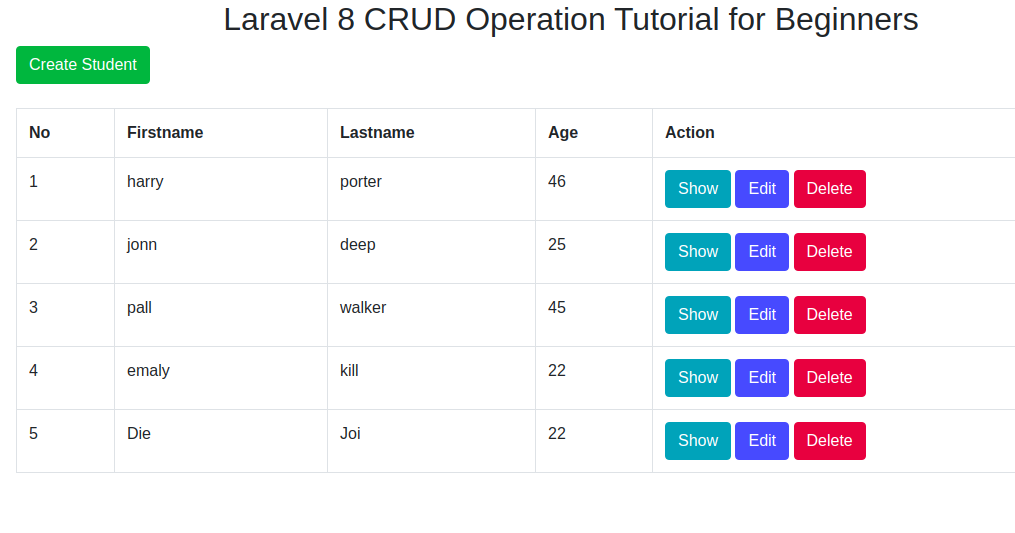
Add Student
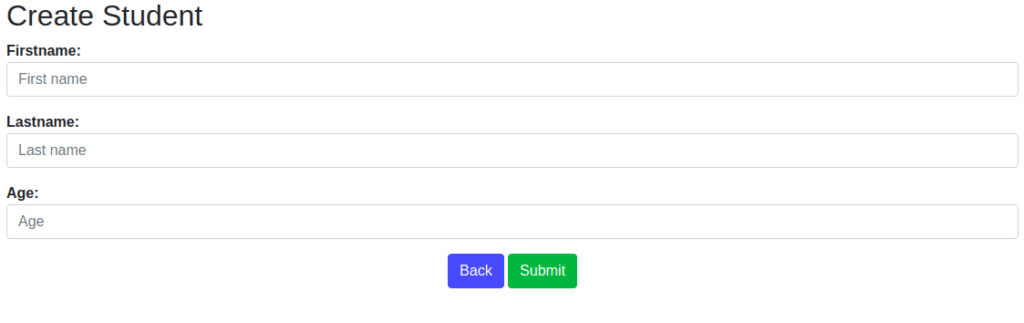
Edit Student
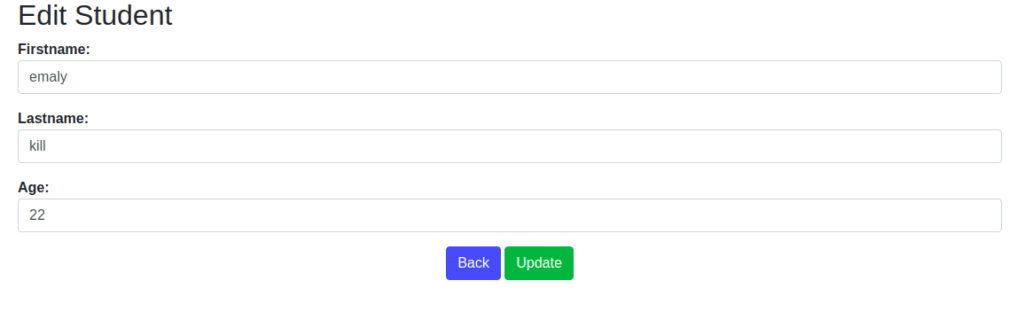
I try to follow your step by step but after run it show error
“Illuminate\Contracts\Container\BindingResolutionException
Target class [StudentController] does not exist.”
How can i fix it.
Add web.php file : use App\Http\Controllers\StudentController;
And replace : Route::resource(‘students’, StudentController::class);
i added web.php file still i am getting erroe like this
use App\Http\Controllers\StudentController; Route::resource(‘students’, StudentController::class);
Please send detail [email protected]