Laravel 8 Custom Forgot & Reset Password Example
In this tutorial, we will learn Laravel 8 Custom Forgot & Reset Password Example step by step explain custom password reset in Laravel 8.. We will explain Laravel custom reset password send an email. We use Laravel it’s own forget password functionality to send reset password links.
I would like to share the Laravel 8 Custom Forgot & Reset Password Example. In this tutorial, you will learn Laravel 8 Custom Forgot & Reset Password Example. And if you want to see an example of how to create Laravel 8 Custom Forgot & Reset Password functionality, you are in the right place. In this tutorial, We created a basic example of the Laravel 8 Custom Forgot & Reset Password Example.
Now we want to create your custom forget password functionality it will help to create a custom reset password function in Laravel 8.
You can see bellow login page add forget password link:
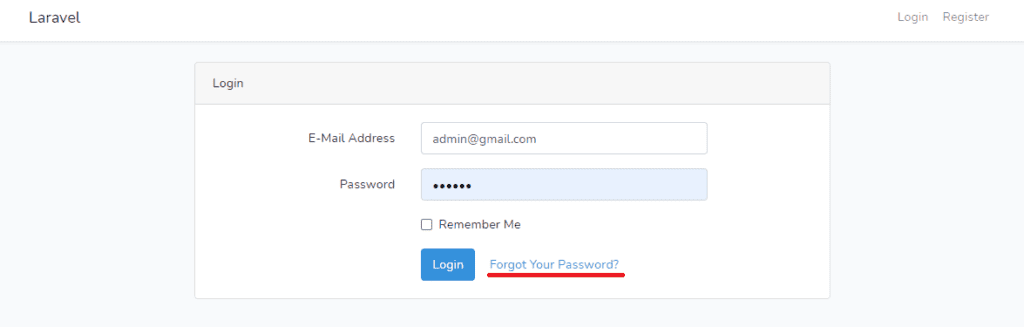
Table of Contents
- Install Laravel
- Check password_resets table
- Create Route
- Add Controller
- Email Configure
- Create Blade Files
Install Laravel
First, we create a fresh Laravel 8 application using the below command. Now, open your command prompt and run the below command:
composer create-project --prefer-dist laravel/laravel blog
Check password_resets table
Laravel default creates password_resets table, and password_resets table not created you add like bellow command and code:
php artisan make:migration create_password_resets_table
<?php #database\migrations\2021_09_09_100000_create_password_resets_table.php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreatePasswordResetsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('password_resets', function (Blueprint $table) { $table->string('email')->index(); $table->string('token'); $table->timestamp('created_at')->nullable(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('password_resets'); } }
Create Route
Now, we can create a custom route for forgetting and reset links.
<?php #routes/web.php use Illuminate\Support\Facades\Route; use App\Http\Controllers\Auth\ForgotPasswordController; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::get('forget-password', [ForgotPasswordController::class, 'ForgetPassword'])->name('ForgetPasswordGet'); Route::post('forget-password', [ForgotPasswordController::class, 'ForgetPasswordStore'])->name('ForgetPasswordPost'); Route::get('reset-password/{token}', [ForgotPasswordController::class, 'ResetPassword'])->name('ResetPasswordGet'); Route::post('reset-password', [ForgotPasswordController::class, 'ResetPasswordStore'])->name('ResetPasswordPost');
Add Controller
Laravel default provides ForgotPasswordController. And we can Simply put the below code.
<?php #app\Http\Controllers\Auth\ForgotPasswordController.php namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use Illuminate\Foundation\Auth\SendsPasswordResetEmails; use Illuminate\Http\Request; use DB; use Carbon\Carbon; use App\Models\User; use Mail; use Hash; use Illuminate\Support\Str; class ForgotPasswordController extends Controller { /* |-------------------------------------------------------------------------- | Password Reset Controller |-------------------------------------------------------------------------- | | This controller is responsible for handling password reset emails and | includes a trait which assists in sending these notifications from | your application to your users. Feel free to explore this trait. | */ use SendsPasswordResetEmails; public function ForgetPassword() { return view('auth.forget-password'); } public function ForgetPasswordStore(Request $request) { $request->validate([ 'email' => 'required|email|exists:users', ]); $token = Str::random(64); DB::table('password_resets')->insert([ 'email' => $request->email, 'token' => $token, 'created_at' => Carbon::now() ]); Mail::send('auth.forget-password-email', ['token' => $token], function($message) use($request){ $message->to($request->email); $message->from(env('MAIL_FROM_ADDRESS'), env('APP_NAME')); $message->subject('Reset Password'); }); return back()->with('message', 'We have emailed your password reset link!'); } public function ResetPassword($token) { return view('auth.forget-password-link', ['token' => $token]); } public function ResetPasswordStore(Request $request) { $request->validate([ 'email' => 'required|email|exists:users', 'password' => 'required|string|min:8|confirmed', 'password_confirmation' => 'required' ]); $update = DB::table('password_resets')->where(['email' => $request->email, 'token' => $request->token])->first(); if(!$update){ return back()->withInput()->with('error', 'Invalid token!'); } $user = User::where('email', $request->email)->update(['password' => Hash::make($request->password)]); // Delete password_resets record DB::table('password_resets')->where(['email'=> $request->email])->delete(); return redirect('/login')->with('message', 'Your password has been successfully changed!'); } }
Email Configure
We will add email Configure on the .env file, and we will send an email to reset the password link from the controller:
#.env MAIL_MAILER=smtp MAIL_HOST=smtp.gmail.com MAIL_PORT=587 [email protected] MAIL_PASSWORD=******* MAIL_ENCRYPTION=tls [email protected] MAIL_FROM_NAME="${APP_NAME}"
Create Blade Files
Now, we will create blade files for the layout, home, login, and register page.
resources/views/auth/layout.blade.php
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- CSRF Token -->
<meta name="csrf-token" content="{{ csrf_token() }}">
<title>{{ config('app.name', 'Laravel') }}</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css" crossorigin="anonymous">
<style type="text/css">
@import url(https://fonts.googleapis.com/css?family=Raleway:300,400,600);
body{
margin: 0;
font-size: .9rem;
font-weight: 400;
line-height: 1.6;
color: #212529;
text-align: left;
background-color: #f5f8fa;
}
.navbar-laravel {
box-shadow: 0 2px 4px rgba(0,0,0,.04);
}
.navbar-brand , .nav-link, .my-form, .login-form {
font-family: Raleway, sans-serif;
}
.my-form {
padding-top: 1.5rem;
padding-bottom: 1.5rem;
}
.my-form .row {
margin-left: 0;
margin-right: 0;
}
.login-form {
padding-top: 1.5rem;
padding-bottom: 1.5rem;
}
.login-form .row {
margin-left: 0;
margin-right: 0;
}
</style>
</head>
<body>
<nav class="navbar navbar-expand-lg navbar-light navbar-laravel">
<div class="container">
<a class="navbar-brand" href="#">Laravel</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarSupportedContent">
<ul class="navbar-nav ml-auto">
@guest
<li class="nav-item">
<a class="nav-link" href="{{ route('login') }}">Login</a>
</li>
<li class="nav-item">
<a class="nav-link" href="{{ route('register') }}">Register</a>
</li>
@else
<li class="nav-item">
<a class="nav-link" href="{{ route('logout') }}">Logout</a>
</li>
@endguest
</ul>
</div>
</div>
</nav>
@yield('content')
</body>
</html>
resources\views\auth\forget-password.blade.php
@extends('auth.layout')
@section('content')
<main class="login-form">
<div class="cotainer">
<div class="row justify-content-center">
<div class="col-md-8">
<div class="card">
<div class="card-header">Reset Password</div>
<div class="card-body">
@if (Session::has('message'))
<div class="alert alert-success" role="alert">
{{ Session::get('message') }}
</div>
@endif
<form action="{{ route('ForgetPasswordPost') }}" method="POST">
@csrf
<div class="form-group row">
<label for="email_address" class="col-md-4 col-form-label text-md-right">E-Mail Address</label>
<div class="col-md-6">
<input type="text" id="email_address" class="form-control" name="email" required autofocus>
@if ($errors->has('email'))
<span class="text-danger">{{ $errors->first('email') }}</span>
@endif
</div>
</div>
<div class="col-md-6 offset-md-4">
<button type="submit" class="btn btn-primary">
Send Reset Password Link
</button>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
</main>
@endsection
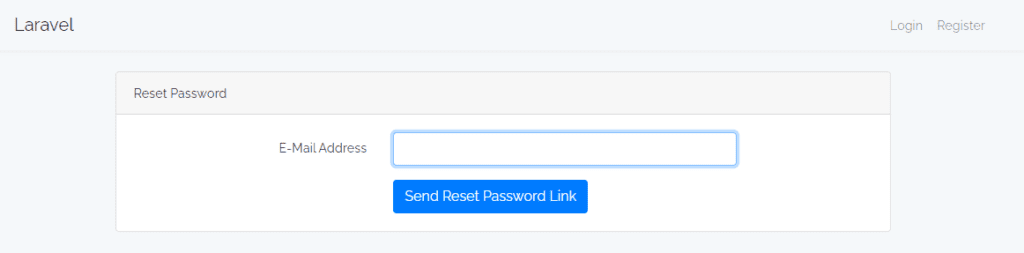
resources\views\auth\forget-password-link.blade.php
@extends('auth.layout')
@section('content')
<main class="login-form">
<div class="cotainer">
<div class="row justify-content-center">
<div class="col-md-8">
<div class="card">
<div class="card-header">Reset Password</div>
<div class="card-body">
<form action="{{ route('ResetPasswordPost') }}" method="POST">
@csrf
<input type="hidden" name="token" value="{{ $token }}">
<div class="form-group row">
<label for="email_address" class="col-md-4 col-form-label text-md-right">E-Mail Address</label>
<div class="col-md-6">
<input type="text" id="email_address" class="form-control" name="email" required autofocus>
@if ($errors->has('email'))
<span class="text-danger">{{ $errors->first('email') }}</span>
@endif
</div>
</div>
<div class="form-group row">
<label for="password" class="col-md-4 col-form-label text-md-right">Password</label>
<div class="col-md-6">
<input type="password" id="password" class="form-control" name="password" required autofocus>
@if ($errors->has('password'))
<span class="text-danger">{{ $errors->first('password') }}</span>
@endif
</div>
</div>
<div class="form-group row">
<label for="password-confirm" class="col-md-4 col-form-label text-md-right">Confirm Password</label>
<div class="col-md-6">
<input type="password" id="password-confirm" class="form-control" name="password_confirmation" required autofocus>
@if ($errors->has('password_confirmation'))
<span class="text-danger">{{ $errors->first('password_confirmation') }}</span>
@endif
</div>
</div>
<div class="col-md-6 offset-md-4">
<button type="submit" class="btn btn-primary">
Reset Password
</button>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
</main>
@endsection
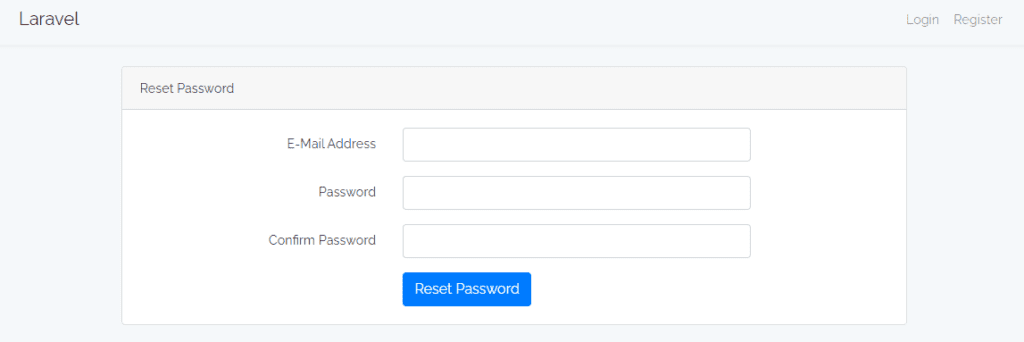
resources\views\auth\forget-password-email.blade.php
<h1>Forget Password Email</h1>
You can reset password from bellow link:
<a href="{{ route('ResetPasswordGet', $token) }}">Reset Password</a>
resources\views\auth\login.blade.php
<div class="form-group row mb-0"> <div class="col-md-8 offset-md-4"> <button type="submit" class="btn btn-primary"> {{ __('Login') }} </button> <a class="btn btn-link" href="{{ route('ForgetPasswordGet') }}"> {{ __('Forgot Your Password?') }} </a> </div> </div>
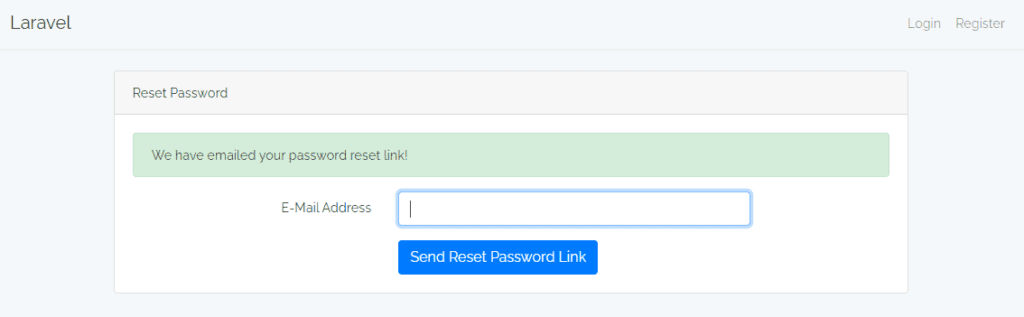
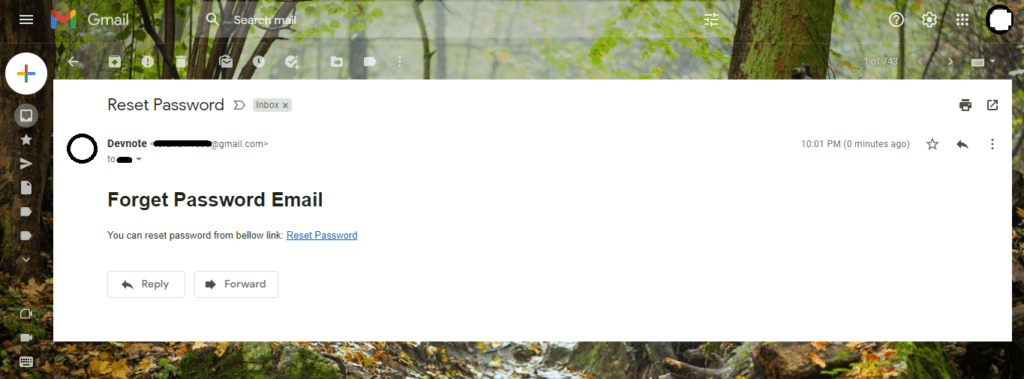
Good tutorial.
Thank you.