Laravel Facebook Login with Socialite Step by Step Guide
We will show you each things step by step an easy way. Just follow few steps and learn how to integrate Facebook social login with laravel.
Step 1 : Install Socialite Package
composer require laravel/socialite
Now open config/app.php file and add service provider and alias.
config/app.php
'providers' => [
....
Laravel\Socialite\SocialiteServiceProvider::class,
],
'aliases' => [
....
'Socialite' => Laravel\Socialite\Facades\Socialite::class,
],
Step 2 : Create Facebook App
Create from here : https://developers.facebook.com/apps and after create account you can copy client id and secret.
Open config/services.php and .env file set id and secret this way:
#config/services.php
return [
....
'facebook' => [
'client_id' => env('FACEBOOK_CLIENT_ID'),
'client_secret' => env('FACEBOOK_CLIENT_SECRET'),
'redirect' => env('FACEBOOK_CALLBACK_URL'),
],
]
Get secret id and secret key from facebook.
We need to required CLIENT ID and CLIENT SECRET to add social Facebook login button in Laravel based project, Lets go to https://developers.facebook.com/apps and create a new app.
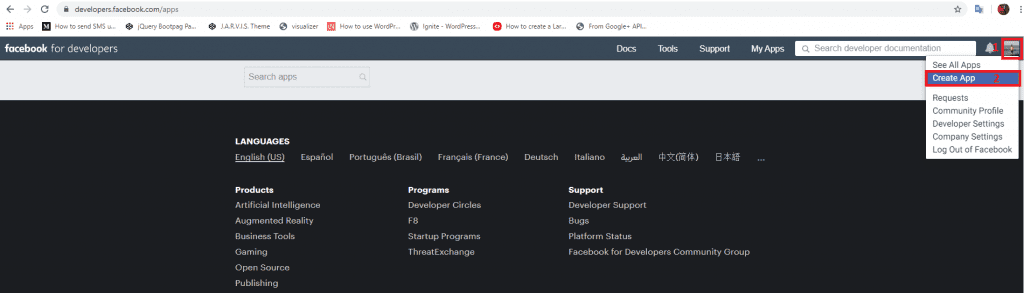
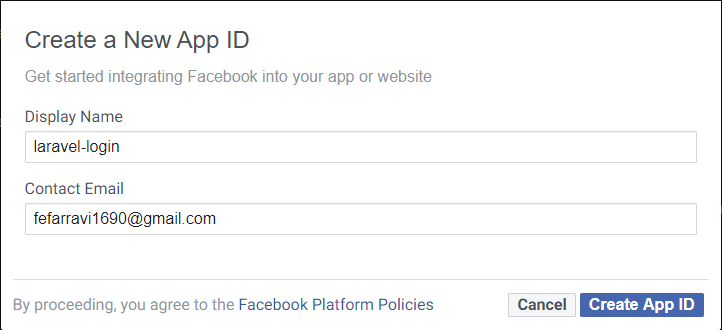
After create the facebook app, go to setting->advanced and set redirect url like below :
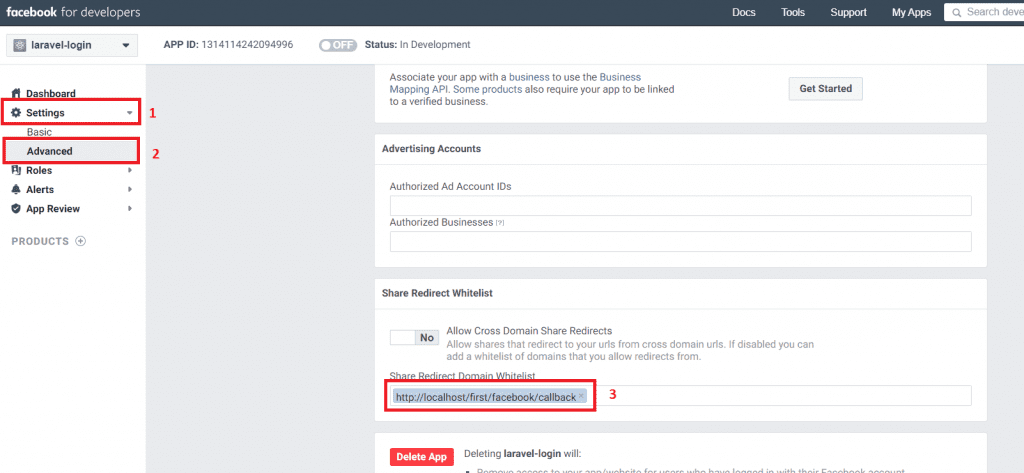
Copy App ID and App SECRET.
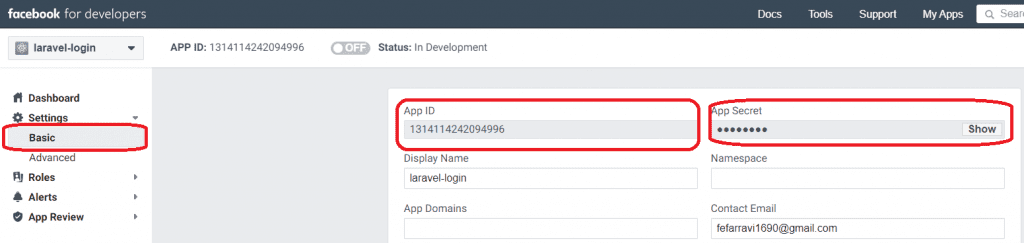
.env file in facebook client and secret id add
FACEBOOK_CLIENT_ID=xxxxxxxxx
FACEBOOK_CLIENT_SECRET=xxxxxxx
FACEBOOK_CALLBACK_URL=http://yourdomain.com/auth/facebook/callback
Step 3 : Create Migration and Model
Add facebook_id in your user table. now create new migration:
Migration :
php artisan make:migration add_socialite_facebook_id_to_users_table
#database/migrations/2020_02_25_063436_add_socialite_facebook_id_to_users_table.php
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class AddNewColunmUsersTable extends Migration
{
public function up()
{
Schema::table("users", function (Blueprint $table) {
$table->string('facebook_id')->nullable();
});
}
public function down()
{
Schema::table("users", function (Blueprint $table) {
$table->dropColumn('facebook_id');
});
}
}
After run this command:
php artisan migrate
#app/User.php
namespace App;
use Illuminate\Notifications\Notifiable;
use Illuminate\Foundation\Auth\User as Authenticatable;
class User extends Authenticatable
{
use Notifiable;
protected $fillable = [
'name', 'email', 'password', 'facebook_id'//change
];
protected $hidden = [
'password', 'remember_token',
];
public function addNew($input)//change
{
$check = static::where('facebook_id',$input['facebook_id'])->first();
if(is_null($check)){
return static::create($input);
}
return $check;
}
}
Step 4 : Routes Define
#routes/web.php
Route::get('/facebook', 'Auth\FacebookController@redirectToFacebook');
Route::get('/facebook/callback', 'Auth\FacebookController@handleFacebookCallback');
Step 5 : Create a controller
#app/Http/Controllers/Auth/FacebookController.php
namespace App\Http\Controllers\Auth;
use App\User;
use App\Http\Controllers\Controller;
use Socialite;
use Exception;
use Auth;
class FacebookController extends Controller
{
public function redirectToFacebook()
{
return Socialite::driver('facebook')->redirect();
}
public function handleFacebookCallback()
{
try {
$user = Socialite::driver('facebook')->user();
$create['name'] = $user->getName();
$create['email'] = $user->getEmail();
$create['facebook_id'] = $user->getId();
$userModel = new User;
$createdUser = $userModel->addNew($create);
Auth::loginUsingId($createdUser->id);
return redirect()->route('home');
} catch (Exception $e) {
return redirect('auth/facebook');
}
}
}
Step 6 : Showing User Name and Avatar
#resources\views\layouts\app.blade.php
<li class="nav-item dropdown">
<a id="navbarDropdown" class="nav-link dropdown-toggle" href="#" role="button" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false" v-pre>
@if(auth()->user()->avatar)
<img src="{{ auth()->user()->avatar }}" alt="avatar" width="32" height="32" style="margin-right: 8px;">
@endif
{{ auth()->user()->name }} <span class="caret"></span>
</a>
<div class="dropdown-menu dropdown-menu-right" aria-labelledby="navbarDropdown">
<a class="dropdown-item" href="{{ route('logout') }}"
onclick="event.preventDefault();
document.getElementById('logout-form').submit();">
{{ __('Logout') }}
</a>
<form id="logout-form" action="{{ route('logout') }}" method="POST" style="display: none;">
@csrf
</form>
</div>
</li>
Ok, now you are ready to use open your browser and check here : URL + ‘/facebook’.