WordPress REST API – Create, Update or Delete posts using Basic Auth
In this tutorial we will learn WordPress REST API – Create, Update or Delete posts using Basic Auth. First of all, we will use JSON basic authentication
a plugin install. Also, You have to download a plugin from GitHub (The link from the official WordPress documentation page). JSON Basic Authentication plugin installed, then we can create step-by-step examples.

Also read: WordPress begins with REST API – Display other’s blogs latest posts
Create a post API
We can use wp_remote_post()
function in the code. wp_remote_post is a WordPress function, so we have to insert it somewhere inside the WP environment. Now, I will create create-post-api.php
a file in the WordPress root directory and also add it at the beginning of the file require('wp-load.php');
.
Example
<?php
#create-post-api.php
require('wp-load.php');
$api_response = wp_remote_post('http://localhost/learn_wordpress/wp-json/wp/v2/posts', array(
'headers' => array(
// 'Authorization' => 'Basic ' . base64_encode('USERNAME:PASSWORD')
'Authorization' => 'Basic ' . base64_encode('admin:admin')
),
'body' => array(
'title' => 'Lorem ipsum dolor sit amet',
'status' => 'draft', // We do not want to publish it immediately
'content' => 'Lorem ipsum dolor sit amet, consectetur adipisicing elit. Corrupti nisi accusantium voluptatibus enim tempore nihil ea atque voluptatum labore alias, dolorem, et neque dolorum eligendi veritatis ipsa eaque repellendus officia.',
'categories' => 16, // category ID
'tags' => '2,3,6', // comma separated
'date' => '2022-07-22T10:00:00', // YYYY-MM-DDTHH:MM:SS
'excerpt' => 'Lorem ipsum dolor sit amet, consectetur adipisicing elit.',
'password' => '123456',
'slug' => 'lorem-ipsum-dolor-sit-amet'
)
));
$body = json_decode( $api_response['body'] );
if(wp_remote_retrieve_response_message( $api_response ) === 'Created') {
$return = array(
'message' => 'The post ' . $body->title->rendered . ' has been created successfully',
);
return wp_send_json($return, 200);
}
Postman Example
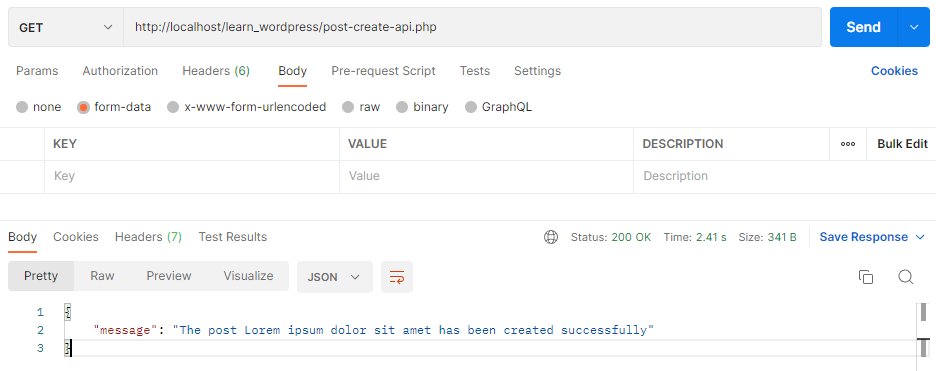
Output

Update a post API
Just update the title of the created post. Here, you replace {POST_ID} with the ID of the post. I will create update-post-api.php
file in the WordPress root directory and also add at the beginning of the file require('wp-load.php');
.
Example
<?php
#update-post-api.php
require('wp-load.php');
$post_id = $_REQUEST['post_id'];
$api_response = wp_remote_post('http://localhost/learn_wordpress/wp-json/wp/v2/posts/'.$post_id, array(
'headers' => array(
'Authorization' => 'Basic ' . base64_encode( 'admin:admin' )
),
'body' => array(
'title' => 'Lorem ipsum dolor sit amet title change'
)
) );
$body = json_decode($api_response['body']);
if(wp_remote_retrieve_response_message( $api_response ) === 'OK') {
$return = array(
'message' => 'The post ' . $body->title->rendered . ' has been updated successfully',
);
return wp_send_json($return, 200);
}
Postman Example
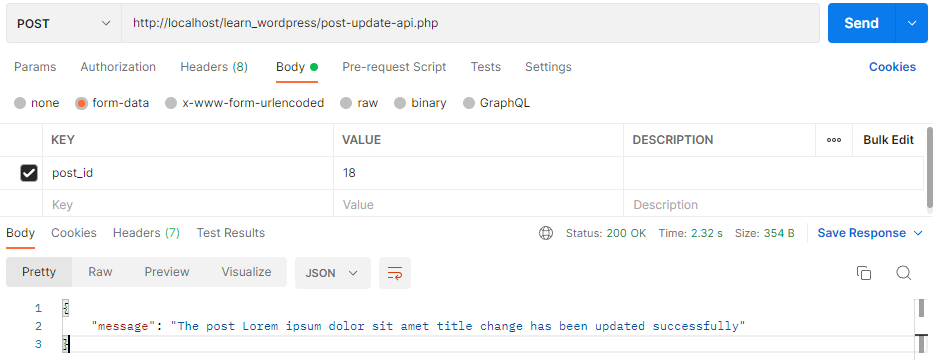
Output

Delete a Post
Now we remove a post from the admin panel. So we add ?force=true at the end of the request, URI and the post will be removed permanently(without moving to the trash).
Example
<?php
#delete-post-api.php
require('wp-load.php');
$post_id = $_REQUEST['post_id'];
$api_response = wp_remote_post('http://localhost/learn_wordpress/wp-json/wp/v2/posts/'.$post_id, array(
'method' => 'DELETE',
'headers' => array(
'Authorization' => 'Basic ' . base64_encode('admin:admin')
)
));
$body = json_decode($api_response['body']);
if( wp_remote_retrieve_response_message( $api_response ) === 'OK' ) {
if( $body->deleted == true ) {
$return = array(
'message' => 'The post ' . $body->previous->title->rendered . ' has been completely deleted'
);
return wp_send_json($return, 200);
} else {
$return = array(
'message' => 'The post ' . $body->title->rendered . ' has been moved to trash'
);
return wp_send_json($return, 200);
}
}
Postman Example
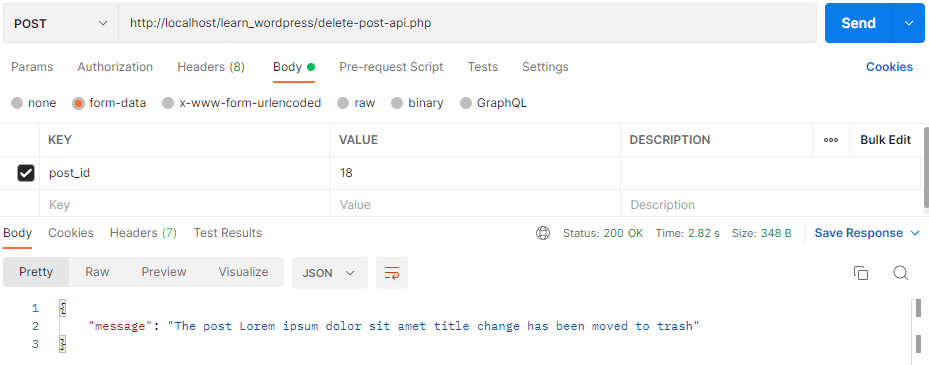
Output
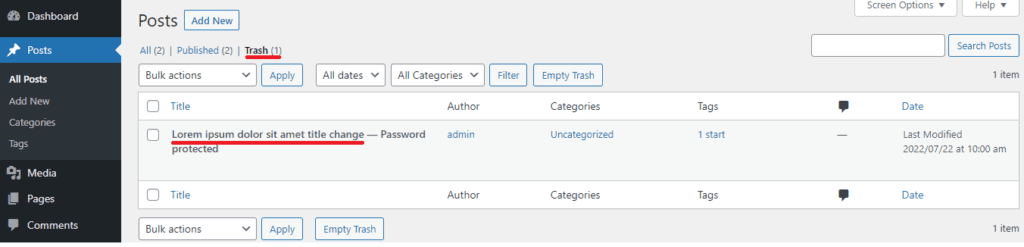
This was a small tutorial on Basic Authentication in WordPress REST API.