How to upload a file AWS S3 using PHP
Today we will discuss uploading a file using PHP with S3.Amazon S3 (Simple Storage Service) provides object storage through a web service interface. Amazon S3 has a simple web services interface that you can use to store and store any amount of data, at any time, from anywhere on the web. Amazon S3 uses the same scalable storage infrastructure and it was created by Amazon.com uses to run its global e-commerce network.
Now first download the AWS SDK for PHP via composer.
Note: If you still don’t have a composer in your windows system, first download it here: https://getcomposer.org/download/
Then, run the below command:
composer require aws/aws-sdk-php
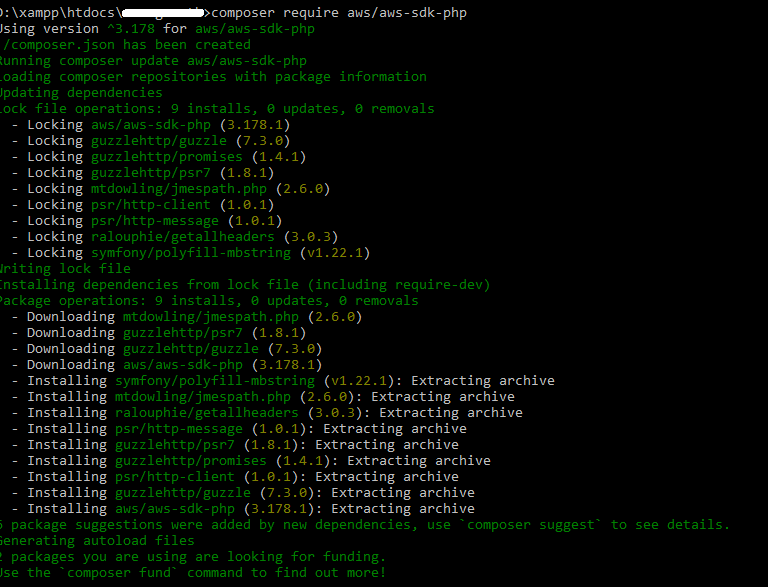
Create a sample PHP file(upload.php) and put the code.
Example
<?php
#upload.php
// Include the SDK
require 'vendor/autoload.php';
$s3Client = new Aws\S3\S3Client([
'region' => 'your_region',
'version' => 'latest',
'credentials' => [
'key' => "access_key_id",
'secret' => "secret_access_key",
]
]);
// Send a putObject.
$keyName = 'devnote.png';
$result = $s3Client->putObject([
'Bucket' => 'bucket_name',
'Key' => $keyName,
'Body' => 'Devnote tutorial!',
//'SourceFile' => 'd:\test.png' //use you want to upload a file from a local computer location.
]);
// Print the body of the result
var_dump($result);
?>
Output
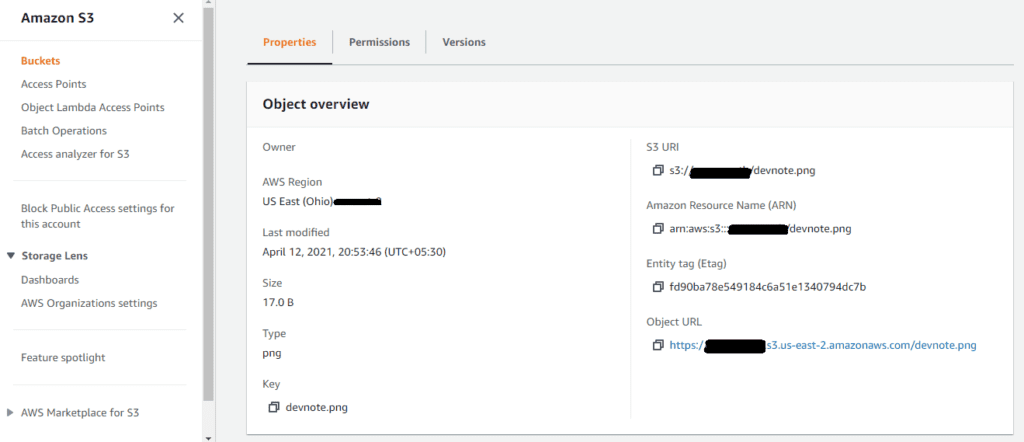
Now you can be able to access the test page via browser and be able to see the file on the S3 target location.
Example
<?php
#upload.php
if(isset($_FILES['image'])){
$file_name = $_FILES['image']['name'];
$temp_file = $_FILES['image']['tmp_name'];
require 'vendor/autoload.php';
$s3Client = new Aws\S3\S3Client([
'region' => 'your_region',
'version' => 'latest',
'credentials' => [
'key' => "access_key_id",
'secret' => "secret_access_key",
]
]);
$result = $s3Client->putObject([
'Bucket' => 'bucket_name',
'Key' => $file_name,
'SourceFile' => $temp_file
]);
var_dump($result);
}
?>
<form method="POST" action="<?php $_SERVER['PHP_SELF']; ?>" enctype="multipart/form-data">
<input type="file" name="image" id="upload_image" />
<input type="submit" value="Submit"/>
</form>
Output

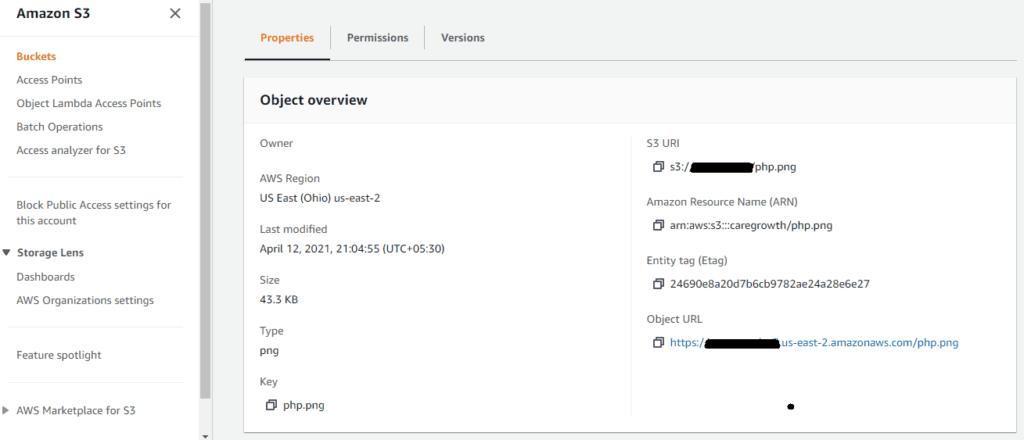