How to Check Disk Space In Laravel
This tutorial is for How to check Disk Space In Laravel. many times we require to check the disk space of our server and we are check manually in Cpanel. if disk space is fully occupied or not, so we will discuss in this tutorial how to check disk space using Laravel. so you can directly check without a login panel or other panel.
Also read: How to check RAM and CPU in Laravel
PHP built-in provides a function to check the free space of the server and total space. Now we will use disk_total_space()
functions.
The first step you need to create a Controller for the logical part. we have created one controller and created check_disk_space()
function.
<?php #app/Http/Controllers/HomeController namespace App\Http\Controllers; use Illuminate\Http\Request; class HomeController extends Controller { public function check_disk_space() { $total_disk = disk_total_space('/'); $total_disk_size = $total_disk / 1073741824; $free_disk = disk_free_space('/'); $used_disk = $total_disk - $free_disk; $disk_used_size = $used_disk / 1073741824; $use_disk = round(100 - (($disk_used_size / $total_disk_size) * 100)); $diskuse = round(100 - ($use_disk)) . '%'; return view('detail_display',compact('diskuse','total_disk_size','disk_used_size')); } }
Then put the below code in your detail_display.blade.php file.
#resources/views/detail_display.blade.php
<html>
<head>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<title>How to check disk space in laravel</title>
</head>
<body class="text-center mt-5">
<h2 class="mb-5">How to check disk space in laravel</h2>
<div class="row">
<div class="col-md-6 offset-md-3">
<h5>Disk Space</h5>
<div class="progress progress-micro mb-10">
<div class="progress-bar bg-indigo-500" style="width: {{$diskuse}}">
<span class="sr-only">{{$diskuse}}</span>
</div>
</div>
<span class="pull-right">{{round($disk_used_size,2)}} GB / {{round($total_disk_size,2)}} GB ({{$diskuse}})</span>
</div>
</div>
</body>
</html>
Finally, route add.
#routes/web.php Route::get('check_disk_space', 'HomeController@check_disk_space');
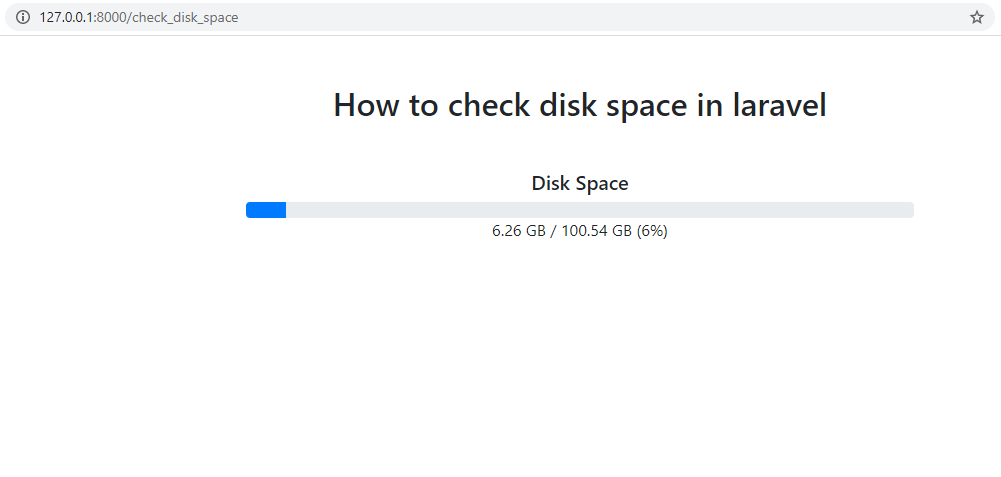