Laravel 8 multiple images upload Tutorial
In this tutorial, we will read How to upload multiple images in Laravel 8. We will upload multiple images with validation using Laravel 8. we will use Laravel image validation, store it in the folder, and add it to the database for Laravel 8 multiple image upload.
Create multiple image uploads using Laravel 8. So basically use this code on your Laravel 8 application. We start from starch first we will upload multiple, store on the server, and store database. Now we will create uploadfiles table using Laravel migration.
Create Laravel 8 multiple File Upload with Validation Example.
Also read: How to display the storage folder image in Laravel?
Table of Contents
- Install fresh Laravel application
- Create Migration and Model
- Create Controller
- Define Routes
- Create Blade File
Install fresh Laravel application
In the first step, we will install Laravel 8 application by typing the following command.
composer create-project --prefer-dist laravel/laravel blog
Create Migration and Model
Here, we need to create database migration for uploadfiles table, and also we will create model files.
php artisan make:model Uploadfile -m
<?php #database\migrations\2021_09_05_094530_create_uploadfiles_table.php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreateUploadfilesTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('uploadfiles', function (Blueprint $table) { $table->id(); $table->string('images'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('uploadfiles'); } }
And migrate uploadfiles table using below command:
php artisan migrate
<?php #app\Models\Uploadfile.php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Uploadfile extends Model { use HasFactory; protected $table = "uploadfiles"; protected $fillable = ['images']; }
Create Controller
We will create UploadController with creating and store method. First, make sure you need to create a storage link. If you do not create a storage link so first run the below command to create a storage folder inside the public folder.
php artisan storage:link Output: The [D:\xampp\htdocs\devnote\public\storage] link has been connected to [D:\xampp\htdocs\devnote\storage\app/public]. The links have been created.
php artisan make:controller UploadController
<?php #app\Http\Controllers\UploadController.php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Uploadfile; class UploadController extends Controller { public function create() { return view('images.create'); } public function store(Request $request) { $this->validate($request, [ 'images' => 'required', 'images.*' => 'mimes:png,jpeg,jpg' ]); if($request->hasfile('images')) { foreach($request->file('images') as $file) { $filename = time() . '_' . $file->getClientOriginalName(); $file->storeAs('public/product_images', $filename); $data[] = $filename; } } $file= new Uploadfile(); $file->images = json_encode($data); $file->save(); return back()->with('success', 'Your images has been successfully uploaded!'); } }
Also read: Laravel storage folder in image upload and old image delete
Define Routes
Now, we will create routes for multiple file uploads.
#routes/web.php
Route::get('file', [App\Http\Controllers\UploadController::class, 'create'])->name('file');
Route::post('file-post', [App\Http\Controllers\UploadController::class, 'store'])->name('file.post');
Create Blade File
Now, we need to create a create.blade.php file in the resources folder in images.
resources/views/images/create.blade.php
<html lang="en">
<head>
<title>Laravel 8 multiple image upload Tutorial</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
</head>
<body>
<div class="container lst">
<h1>Laravel 8 multiple image upload Tutorial</h1>
@if (count($errors) > 0)
<div class="alert alert-danger">
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
@if(session('success'))
<div class="alert alert-success">
{{ session('success') }}
</div>
@endif
<form method="post" action="{{ route('file.post') }}" enctype="multipart/form-data">
{{csrf_field()}}
<div class="control-group">
<input type="file" name="images[]" class="myfrm form-control" multiple>
</div>
<button type="submit" class="btn btn-success" style="margin-top:10px">Upload</button>
</form>
</div>
</body>
</html>
Now you check http://127.0.0.1:8000/file
.
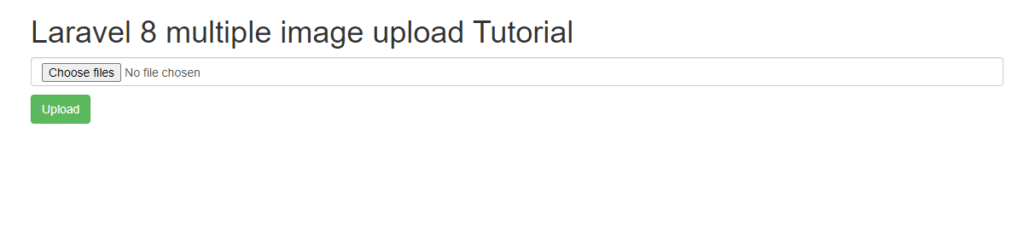